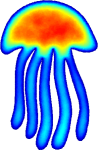 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
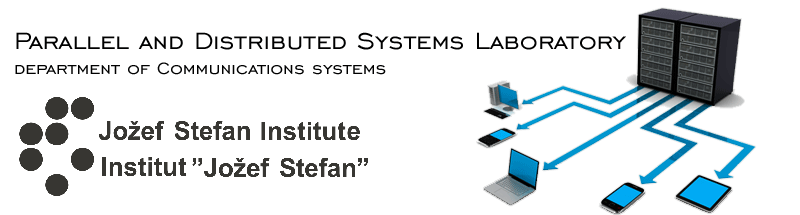 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_SHAPESTORAGE_FWD_HPP_
2 #define MEDUSA_BITS_OPERATORS_SHAPESTORAGE_FWD_HPP_
19 template <
class shape_storage_type>
20 class ExplicitOperators;
22 template <
class shape_storage_type>
23 class ExplicitVectorOperators;
25 template <
class shape_storage_type,
class matrix_type,
class rhs_type>
26 class ImplicitOperators;
28 template <
class shape_storage_type,
class matrix_type,
class rhs_type>
29 class ImplicitVectorOperators;
39 template <
typename Derived,
typename vec_t,
typename OpFamilies =
40 std::tuple<Lap<vec_t::dim>, Der1s<vec_t::dim>, Der2s<vec_t::dim>>>
49 constexpr
static int num_operators = std::tuple_size<op_families_tuple>::value;
64 template <
typename T>
SINL T*
access(std::vector<T>& v,
int op,
int node)
const {
65 return static_cast<const Derived*
>(
this)->
access(v, op, node); }
67 template <
typename T>
SINL T*
access(std::vector<T>& v,
int node)
const {
70 template <
typename T>
SINL const T*
access(
const std::vector<T>& v,
int op,
int node)
const {
71 return static_cast<const Derived*
>(
this)->
access(v, op, node); }
73 template <
typename T>
SINL const T*
access(
const std::vector<T>& v,
int node)
const {
81 void resize(
const std::vector<int>& support_sizes) {
83 static_cast<Derived*
>(
this)->resize_(support_sizes);
96 SINL Eigen::Map<const Eigen::Matrix<int, Eigen::Dynamic, 1>>
support(
int node)
const;
130 return get<tuple_index<op_family, op_families_tuple>::value>(op, node, j);
140 return get<tuple_index<op_family, op_families_tuple>::value>(node, j);
144 template <
int op_family> Eigen::Map<const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>>
150 template <
typename op_family> Eigen::Map<const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>>
152 return getShape<tuple_index<op_family, op_families_tuple>::value>(op, node);
156 template <
int op_family>
157 SINL Eigen::Map<const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>>
getShape(
int node)
const {
162 template <
typename op_family>
163 SINL Eigen::Map<const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>>
getShape(
int node)
const {
164 return getShape<tuple_index<op_family, op_families_tuple>::value>(node);
169 template <
int op_family>
170 SINL void setShape(
int op,
int node,
const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>& shape) {
171 std::memcpy(
access(
shapes_[op_family], op, node), shape.data(),
176 template <
typename op_family>
177 SINL void setShape(
int op,
int node,
const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>& shape) {
178 return setShape<tuple_index<op_family, op_families_tuple>::value>(op, node, shape);
182 template <
int op_family>
183 SINL void setShape(
int node,
const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>& shape) {
188 template <
typename op_family>
189 SINL void setShape(
int node,
const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>& shape) {
190 return setShape<tuple_index<op_family, op_families_tuple>::value>(node, shape);
199 Eigen::Map<const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>>
laplace(
int node)
const;
202 SINL void setLaplace(
int node,
const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>& shape);
208 Eigen::Map<const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>>
d1(
int var,
int node)
const;
211 SINL void setD1(
int var,
int node,
const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>& shape);
220 Eigen::Map<const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>>
221 d2(
int varmin,
int varmax,
int node)
const;
224 SINL void setD2(
int varmin,
int varmax,
int node,
225 const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>& shape);
235 template <
typename M,
typename R>
238 template <
typename M,
typename R>
242 template <
typename D,
typename V,
typename O>
249 #endif // MEDUSA_BITS_OPERATORS_SHAPESTORAGE_FWD_HPP_
SINL void setD1(int var, int node, const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > &shape)
Sets shape for derivative wrt. variable var for node to shape.
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
int domain_size_
Total number of nodes.
void resize(const std::vector< int > &support_sizes)
Resizes the storage to accommodate shapes of given sizes.
SINL void setShape(int node, const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > &shape)
Set the shape for node for the only operator in op_family (by index).
friend std::ostream & operator<<(std::ostream &os, const ShapeStorage< D, V, O > &shapes)
Output basic info about this shape storage.
@ dim
Number of elements of this matrix.
constexpr static int num_operators
Number of operator families in this storage.
SINL scalar_t d1(int var, int node, int j) const
Return j-th shape coefficient for derivative wrt. variable var in node.
int supportSize(int node) const
Returns support size of node-th node.
ShapeStorage()
Constructs empty storage with size 0. Can be resized with resize.
const SINL T * access(const std::vector< T > &v, int node) const
Returns const pointer to the start of values for node-th node.
Shape storage base class.
SINL scalar_t get(int op, int node, int j) const
Get the weight of j-th stencil node of node for operator op in op_family (by index).
ImplicitOperators< Derived, M, R > implicitOperators(M &matrix, R &rhs) const
Construct implicit operators over this storage.
A class for evaluating typical operators needed in spatial discretization.
SINL T * access(std::vector< T > &v, int node) const
Returns pointer to the start of values for node-th node.
SINL void setLaplace(int node, const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > &shape)
Sets the laplace shape for node to shape.
vec_t::scalar_t scalar_t
Scalar type used.
std::array< Range< scalar_t >, num_operators > shapes_
Tuple of shape containers for given operators.
SINL scalar_t laplace(int node, int j) const
Return j-th laplace shape coefficient for node-th node.
Range< int > support_
Local copy of support domains.
OpFamilies op_families_tuple
Tuple of operator families.
vec_t vector_t
Vector type used.
SINL int support(int node, int j) const
Returns index of j-th neighbour of node-th node, i.e. support[node][j], but possibly faster.
A class for evaluating typical operators needed in spatial discretization.
ExplicitVectorOperators< Derived > explicitVectorOperators() const
Construct explicit vector operators over this storage.
Range< int > supportSizes() const
Returns a vector of support sizes for all nodes, useful for matrix space preallocation.
This class represents implicit operators that fill given matrix M and right hand side rhs with approp...
Eigen::Map< const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > > getShape(int op, int node) const
Get the shape for node for operator op in op_family (by index).
void setSupport(int node_idx, const std::vector< int > &support)
Sets support of node-th node to support.
SINL void setD2(int varmin, int varmax, int node, const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > &shape)
Sets shape for mixed derivative wrt. variables varmin and varmax for node to shape.
const std::array< Range< scalar_t >, num_operators > & shapes() const
Read access to complete shape storage.
Range< int > supportSizesVec() const
Returns a dim*N vector of dim*support_size, useful for matrix space preallocation for vector equation...
size_t memoryUsed() const
Returns the approximate memory used (in bytes).
SINL scalar_t d2(int varmin, int varmax, int node, int j) const
Return j-th shape coefficient for mixed derivative wrt.
#define SINL
Strong inline macro.
friend Derived
Be friends with derived class.
int size() const
Returns number of nodes.
ImplicitVectorOperators< Derived, M, R > implicitVectorOperators(M &matrix, R &rhs) const
Construct implicit vector operators over this storage.
SINL T * access(std::vector< T > &v, int op, int node) const
Returns pointer to the start of values for node-th node for op-th operator.
@ dim
Dimensionality of the domain.
SINL void setShape(int op, int node, const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > &shape)
Set the shape for node for operator op in op_family (by index).
This class represents implicit vector operators that fill given matrix M and right hand side rhs with...
SINL Eigen::Map< const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > > getShape(int node) const
Get the shape for node for the only operator in op_family (by index).
const SINL T * access(const std::vector< T > &v, int op, int node) const
Returns const pointer to the start of values for node-th node for op-th operator.
ExplicitOperators< Derived > explicitOperators() const
Construct explicit operators over this storage.
SINL scalar_t get(int node, int j) const
Get the j-th weight of shape for node for the only operator in op_family (by index).