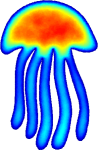 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
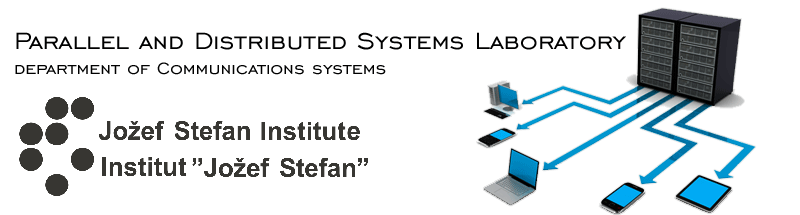 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_EXPLICITOPERATORS_FWD_HPP_
2 #define MEDUSA_BITS_OPERATORS_EXPLICITOPERATORS_FWD_HPP_
34 template <
class shape_storage_type>
38 typedef typename shape_storage_t::vector_t
vector_t;
80 template <
typename scalar_field_t>
82 return apply<Lap<dim>, scalar_field_t>(u, node);
94 template <
typename scalar_field_t>
96 grad(
const scalar_field_t& u,
int node)
const;
138 template <
typename scalar_field_t>
140 const scalar_field_t& u,
int node,
const vector_t& normal,
149 template <
typename scalar_field_t>
151 const scalar_field_t& u,
int var,
int node)
const {
152 return apply<Der1s<dim>, scalar_field_t>(u, node,
Der1<dim>(var));
162 template <
typename scalar_field_t>
164 int varmax,
int node)
const {
165 return apply<Der2s<dim>, scalar_field_t>(u, node,
Der2<dim>(varmin, varmax));
179 template <
typename op_family_t,
typename scalar_field_t>
181 const scalar_field_t& u,
int node,
typename op_family_t::operator_t o)
const;
185 template <
typename op_family_t,
typename scalar_field_t>
187 return apply<op_family_t, scalar_field_t>(u, node, {});
191 template <
typename S>
197 #endif // MEDUSA_BITS_OPERATORS_EXPLICITOPERATORS_FWD_HPP_
const shape_storage_t * ss
Pointer to shape storage, but name is shortened for readability.
shape_storage_t::vector_t vector_t
Vector type.
Root namespace for the whole library.
scalar_type< scalar_field_t >::type lap(const scalar_field_t &u, int node) const
Returns an approximation of Laplacian of field u in node-th node.
Scalar scalar_t
Type of the elements, alias of Scalar.
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
friend std::ostream & operator<<(std::ostream &os, const ExplicitOperators< S > &op)
Output basic information about given operators.
scalar_type< scalar_field_t >::type neumann(const scalar_field_t &u, int node, const vector_t &normal, typename scalar_type< scalar_field_t >::type val) const
Calculates a new field value a for u at node, such that the Neumann boundary condition du/dn(node) = ...
void setShapes(const shape_storage_t &shape_storage_)
Sets a new shape storage from which this operators are generated.
scalar_type< scalar_field_t >::type apply(const scalar_field_t &u, int node) const
Overload for default constructible operator.
@ dim
Number of elements of this matrix.
ExplicitOperators()
Construct empty explicit operators.
A class for evaluating typical operators needed in spatial discretization.
scalar_type< scalar_field_t >::type apply(const scalar_field_t &u, int node, typename op_family_t::operator_t o) const
Returns an approximation of applying the requested operator to field u at node node.
Represents a second derivative wrt. var1 and var2.
shape_storage_t::scalar_t scalar_t
Scalar type.
ExplicitOperators(const shape_storage_t &ss)
Construct explicit operators over given shape storage.
shape_storage_type shape_storage_t
Type of shape storage.
scalar_type< scalar_field_t >::type d2(const scalar_field_t &u, int varmin, int varmax, int node) const
Returns an approximation of requested second derivative of field u in node-th node.
Vec< typename scalar_type< scalar_field_t >::type, ExplicitOperators< shape_storage_type >::dim > grad(const scalar_field_t &u, int node) const
Returns an approximation of gradient of field u in node-th node.
Represents a first derivative wrt. var.
scalar_type< scalar_field_t >::type d1(const scalar_field_t &u, int var, int node) const
Returns an approximation of requested derivative of field u in node-th node.
bool hasShapes() const
Returns true if operators have a non-null pointer to storage and false otherwise.
scalar_field_t::Scalar type
Default scalar type.
@ dim
Dimensionality of the function domain.