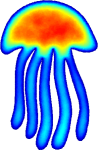 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
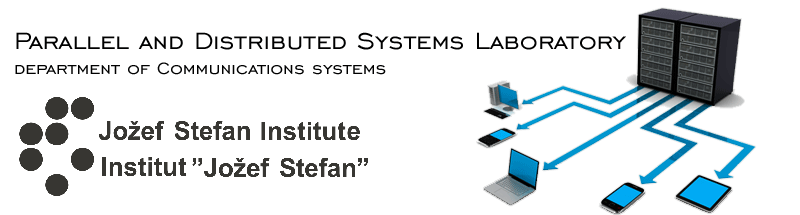 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_IMPLICITOPERATORS_FWD_HPP_
2 #define MEDUSA_BITS_OPERATORS_IMPLICITOPERATORS_FWD_HPP_
39 template <
class shape_storage_type,
class matrix_type,
class rhs_type>
45 typedef typename shape_storage_t::vector_t
vector_t;
46 typedef typename matrix_t::Scalar
scalar_t;
71 template <
typename derived_t>
class OpBase;
97 "Cannot add together terms for different matrix rows %d and %d.",
102 template <
typename derived_t>
118 template <
typename derived_t>
134 return static_cast<const derived_t &
>(*this).eval(alpha);
147 template <
typename other_derived_t>
154 #define USE_BASE(Name) \
156 using OpBase<Name>::op; \
157 using OpBase<Name>::node; \
158 using OpBase<Name>::row; \
159 using OpBase<Name>::OpBase; \
160 friend class ImplicitOperators; \
162 using OpBase<Name>::eval; \
163 using OpBase<Name>::operator=;
175 template <
typename op_family_t>
179 typename op_family_t::operator_t
o;
189 int idx = op_family_t::index(
o);
190 for (
int i = 0; i <
op.
ss->supportSize(
node); ++i) {
192 op.
ss->template get<op_family_t>(idx,
node, i));
212 for (
int i = 0; i <
op.
ss->supportSize(
node); ++i) {
214 for (
int var = 0; var <
op.
dim; ++var) {
356 assert_msg(std::abs(unit_normal.norm() - 1.0) < 1e-13,
357 "Normal %s is not of unit length, got %.16f.", unit_normal, unit_normal.norm());
358 return grad(node, unit_normal, row);
414 return der2(node, varmin, varmax, node);
426 template <
typename op_family_t>
432 template <
typename op_family_t>
434 return apply<op_family_t>(node, o, node);
438 template <
typename op_family_t>
443 template <
typename S,
typename M,
typename R>
449 #endif // MEDUSA_BITS_OPERATORS_IMPLICITOPERATORS_FWD_HPP_
Root namespace for the whole library.
rhs_type rhs_t
Right hand side type.
int row() const
Return absolute index of this row operation.
GradOp grad(int node, const vector_t &v)
Same as grad with row equal to current node.
Class representing the directional derivative (gradient) operation.
const shape_storage_t * ss
Shape storage, but name is shortened for readability.
int row_
Index of the row to which this operation refers.
RowOp eval() const
Eval with alpha = 1.0.
Base class for all elementary implicit operations.
void addToRhs(int i, scalar_t v)
Adds v to rhs[i].
matrix_t::Scalar scalar_t
Scalar type of matrix elements.
shape_storage_t::vector_t vector_t
Vector type.
GradOp grad(int node, const vector_t &v, int row)
Sets implicit equation that gradient of a field at node-th point along v is equal to some value.
RowOp eval(scalar_t alpha) const
Write appropriate coefficients for this operation to the matrix.
@ dim
Number of elements of this matrix.
ImplicitOperators()
Default constructor sets offset to 0 and pointers to nullptr.
rhs_t * rhs
Pointer to right hand side.
RowOp operator+(const RowOp &other) const
Add two row operations together.
GradOp neumann(int node, const vector_t &unit_normal, int row)
Sets neumann boundary conditions in node node.
#define assert_msg(cond,...)
Assert with better error reporting.
bool hasMatrix() const
Returns true if operators have a non-null pointer to problem matrix.
BasicOp< Lap< dim > > lap(int node, int row)
Sets implicit equation that Laplacian of a field at node-th point is equal to some value.
RowOp operator+(RowOp right)
Combine this operation with another row operation.
friend RowOp operator*(scalar_t alpha, const OpBase &o)
Multiply with scalar from the left.
RowOp operator-()
Multiply this operation by -1.
op_family_t::operator_t o
Basic operator with precomputed shape functions.
matrix_t * M
Pointer to problem matrix.
RowOp operator+(const OpBase< other_derived_t > &right)
Combine tho operation with another operation. Both operations are evaluated.
bool hasShapes() const
Returns true if operators have a non-null pointer to storage.
RowOp operator+(const OpBase< derived_t > &right)
Add normal operation to a row operation by evaluating the former and adding normally.
friend std::ostream & operator<<(std::ostream &os, const ImplicitOperators< S, M, R > &op)
Output basic info about given operators.
void setProblem(matrix_t &M, rhs_t &rhs, int row_offset=0, int col_offset=0)
Sets current matrix and right hand side.
@ dim
Dimensionality of the function domain.
BasicOp< op_family_t > apply(int node, typename op_family_t::operator_t o)
Same as apply with row equal to current node.
matrix_type matrix_t
Matrix type.
BasicOp< Der2s< dim > > der2(int node, int varmin, int varmax, int row)
Sets implicit equation that second derivative of a field at node-th point with respect to varmin and...
BasicOp< Der2s< dim > > der2(int node, int varmin, int varmax)
Same as der2 with row equal to current node.
vector_t vec
Vector representing the direction of differentiation.
void setRowOffset(int row_offset)
Sets row offset for given matrix, treating it as is the first row had index row_offset.
void operator=(scalar_t x)
Evaluates *this and sets rhs to x.
ImplicitOperators & op
Reference to underlying operators.
This class represents implicit operators that fill given matrix M and right hand side rhs with approp...
OpBase(ImplicitOperators &op, int node, int row)
Construct operation for a given node.
RowOp(ImplicitOperators &op, int row)
Construct a row operation for a given row.
RowOp eval(scalar_t alpha) const
Evaluate this operator.
BasicOp(ImplicitOperators &op, int node, int row, typename op_family_t::operator_t o)
Construct given basic operator 'o'.
int row
Matrix row to which the operation should be applied (without offset).
BasicOp< Der1s< dim > > der1(int node, int var, int row)
Sets implicit equation that derivative of a field at node-th point with respect to var is equal to s...
void setColOffset(int col_offset)
Sets col offset for given matrix, treating it as is the first column had index col_offset.
int row_offset
Row offset to be used when accessing matrix or rhs coefficients.
ValueOp value(int node, int row)
Sets implicit equation that value of a field is equal to some other value.
BasicOp< Lap< dim > > lap(int node)
Same as lap with row equal to current node.
RowOp eval(scalar_t alpha) const
Evaluate this operator.
bool hasRhs() const
Returns true if operators have a non-null pointer to problem right hand side.
ImplicitOperators(const shape_storage_t &ss)
Set only shape storage, the rest as default constructor.
BasicOp< Der1s< dim > > der1(int node, int var)
Same as der1 with row equal to current node.
void addToM(int i, int j, scalar_t v)
Add v to M(i, j).
BasicOp< op_family_t > apply(int node)
Overload for default-constructible operator.
GradOp neumann(int node, const vector_t &n)
Same as neumann with row equal to current node.
ImplicitOperators & op
Reference to underlying operators.
shape_storage_type shape_storage_t
Type of shape storage.
int col_offset
Column offset to be used when accessing matrix coefficients.
RowOp operator*(scalar_t alpha)
Multiply this operation by a scalar.
Class representing an operation on a specific row of the matrix.
void operator=(scalar_t value)
Assigns given value to the right hand side.
BasicOp< op_family_t > apply(int node, typename op_family_t::operator_t o, int row)
Add the weights for operator o to the appropriate elements in the matrix.
int node
Index of the point for which to apply the operation.
Class representing Basic operators i.e. Der1, Der2, Lap or user defined custom operators.
Class representing the "evaluate" operation, i.e. the zero-th derivative.
ValueOp value(int node)
Same as value with row equal to current node.
#define USE_BASE(Name)
Macro that inherits appropriate members from parent OpBase class.