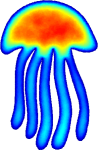 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
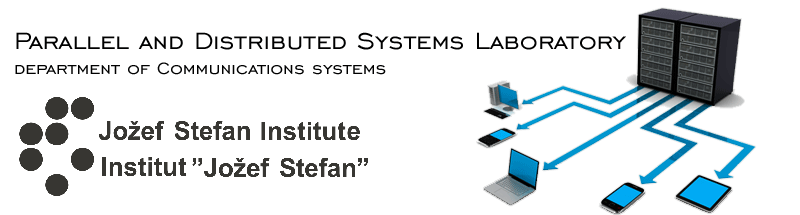 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_IMPLICITVECTOROPERATORS_FWD_HPP_
2 #define MEDUSA_BITS_OPERATORS_IMPLICITVECTOROPERATORS_FWD_HPP_
43 template <
class shape_storage_type,
class matrix_type,
class rhs_type>
57 typedef Eigen::Matrix<scalar_t, dim, 1>
vector_t;
69 assert_msg(v == v,
"You tried to set M(%d, %d) to NAN. Check your computes shapes.",
76 "You tried to set rhs(%d) to NaN. Check you computed shapes.",
row_offset+i);
77 for (
int d = 0; d <
dim; ++d) {
110 "Cannot add together terms for different matrix rows %d and %d.",
115 template <
typename derived_t>
130 template <
typename derived_t>
146 return static_cast<const derived_t &
>(*this).eval(alpha);
159 template <
typename other_derived_t>
166 #define USE_VECTOR_BASE(Name) \
168 using VecOpBase<Name>::op; \
169 using VecOpBase<Name>::node; \
170 using VecOpBase<Name>::row; \
171 using VecOpBase<Name>::VecOpBase; \
172 friend class ImplicitVectorOperators; \
174 using VecOpBase<Name>::eval; \
175 using VecOpBase<Name>::operator=;
182 for (
int d = 0; d <
dim; ++d) {
190 template <
typename op_family_t>
194 typename op_family_t::operator_t
o;
201 int idx = op_family_t::index(
o);
202 for (
int d = 0; d <
dim; ++d) {
203 for (
int i = 0; i <
op.
ss->supportSize(
node); ++i) {
205 alpha *
op.
ss->template get<op_family_t>(idx,
node, i));
227 for (
int i = 0; i <
op.
ss->supportSize(
node); ++i) {
229 for (
int var = 0; var <
dim; ++var) {
232 for (
int d = 0; d <
dim; ++d) {
250 for (
int d = 0; d <
dim; ++d) {
251 for (
int k = 0; k <
op.
ss->supportSize(
node); ++k) {
252 for (
int j = 0; j <
dim; ++j) {
253 int dmin = std::min(d, j);
254 int dmax = std::max(d, j);
257 alpha *
op.
ss->d2(dmin, dmax,
node, k));
280 for (
int i = 0; i <
dim; ++i) {
281 for (
int j = 0; j <
dim; ++j) {
284 for (
int j = 0; j <
dim; ++j) {
301 assert_msg(0 <= idx && idx <
op.
dim,
"Equation number %d out of range [0, %d).",
309 "Dimension %d out of range, expected in range [0, %d).", comp,
dim);
375 assert_msg(0 <= num && num <
dim,
"Equation must be in range [0, %d), got %d", num,
dim);
432 template <
typename op_family_t>
438 template <
typename op_family_t>
440 return apply<op_family_t>(node, o, node);
444 template <
typename op_family_t>
506 assert_msg(std::abs(unit_normal.norm() - 1.0) < 1e-13,
507 "Normal %s is not of unit length, got %.16f.", unit_normal, unit_normal.norm());
508 return grad(node, unit_normal, row);
534 assert_msg(std::abs(unit_normal.norm() - 1.0) < 1e-13,
535 "Normal %s is not of unit length, got %.16f.", unit_normal, unit_normal.norm());
536 return TractionOp(*
this, node, row, lam, mu, unit_normal);
540 return traction(node, lam, mu, n, node);
544 template <
typename S,
typename M,
typename R>
550 #endif // MEDUSA_BITS_OPERATORS_IMPLICITVECTOROPERATORS_FWD_HPP_
RowVecOp eval(scalar_t alpha) const
Evaluate this operator.
GradOp neumann(int node, const domain_vector_t &unit_normal, int row)
Sets neumann boundary conditions in node node.
bool hasMatrix() const
Returns true if operators have a non-null pointer to problem matrix.
ImplicitVectorOperators(const shape_storage_t &ss)
Set only shape storage, the rest as default constructor.
rhs_type rhs_t
Right hand side type.
TractionOp traction(int node, scalar_t lam, scalar_t mu, const domain_vector_t &n)
Same as traction with row equal to current node.
Root namespace for the whole library.
bool hasRhs() const
Returns true if operators have a non-null pointer to problem right hand side.
int row() const
Return absolute index of this row operation.
BasicOp< op_family_t > apply(int node, typename op_family_t::operator_t o)
Same as apply with row equal to current node.
RowVecOp operator+(RowVecOp right)
Combine this operation with another row operation.
RowVecOp eval(scalar_t alpha) const
Write appropriate coefficients for this operation to the matrix.
rhs_t * rhs
Pointer to right hand side.
ValueOp value(int node, int row)
Sets implicit equation that value of a vector field is equal to some other value.
ImplicitVectorOperators & op
Reference to underlying operators.
Equation(ImplicitVectorOperators &op, int idx)
Create an equation with given index.
RowVecOp operator+(const RowVecOp &other) const
Add two row operations together.
void setRowOffset(int row_offset)
Sets row offset for given matrix, treating it as is the first row had index row_offset.
matrix_type matrix_t
Matrix type.
GradOp grad(int node, const domain_vector_t &v, int row)
Sets implicit equation that gradient of a vector field along v is equal to some other value.
void addToM(int i, int j, scalar_t v)
Adds v to M(i, j), i.e. M(i, j) += v.
@ dim
Number of elements of this matrix.
Class representing the "evaluate" operation, i.e. the zero-th derivative.
RowVecOp operator*(scalar_t alpha)
Multiply this operation by a scalar.
RowVecOp eval(scalar_t alpha) const
Evaluate this operator.
TractionOp traction(int node, scalar_t lam, scalar_t mu, const domain_vector_t &unit_normal, int row)
Sets traction boundary conditions in node node.
GradOp neumann(int node, const domain_vector_t &n)
Same as neumann with row equal to current node.
BasicOp(ImplicitVectorOperators &op, int node, int row, typename op_family_t::operator_t o)
Construct given basic operator 'o'.
GradDivOp graddiv(int node)
Same as graddiv with row equal to current node.
friend std::ostream & operator<<(std::ostream &os, const ImplicitVectorOperators< S, M, R > &op)
Output basic info about given operators.
#define assert_msg(cond,...)
Assert with better error reporting.
int eq_ixd
Index of the equation.
Class representing basic operators.
friend RowVecOp operator*(scalar_t alpha, const VecOpBase &op)
Multiply with scalar from the left.
matrix_t * M
Pointer to problem matrix.
@ dim
Dimensionality of the domain.
Eigen::Matrix< scalar_t, dim, 1 > vector_t
Vector type.
Class representing the directional derivative (gradient) operation.
void addToRhs(int i, const vector_t &v)
Adds components of v to i, i+N, ..., i+(dim-1)*N-th rows of rhs.
scalar_t mu
2nd Lame constant.
domain_vector_t normal
Unit normal to the surface where traction os desired.
TractionOp(ImplicitVectorOperators &op, int node, int row, scalar_t lam, scalar_t mu, const domain_vector_t &normal)
Create traction operator with given Lame constants and outside unit normal.
#define USE_VECTOR_BASE(Name)
Macro that inherits appropriate members from parent VecOpBase class.
domain_vector_t vec
Vector representing the direction of differentiation.
void operator=(const vector_t &value)
Assigns given value to the right hand side.
GradOp grad(int node, const domain_vector_t &v)
Same as grad with row equal to current node.
void setProblem(matrix_t &M, rhs_t &rhs, int row_offset=0, int col_offset=0)
Sets current matrix and right hand side.
RowVecOp operator+(const VecOpBase< other_derived_t > &right)
Combine tho operation with another operation. Both operations are evaluated.
Equation eq(int num)
Choose one specific component of the vector equation to write to.
bool hasShapes() const
Returns true if operators have a non-null pointer to storage and false otherwise.
This class represents implicit operators that fill given matrix M and right hand side rhs with approp...
GradDivOp graddiv(int node, int row)
Sets implicit equation that gradient of divergence of a vector field is equal to some other value.
int row
Matrix row to which the operation should be applied (without offset).
void operator=(const vector_t &x)
Evaluates *this and sets rhs to x.
Class representing the gradient of the divergence operator, i.e. operator .
const shape_storage_t * ss
Shape storage, but name is shortened for readability.
RowVecOp(ImplicitVectorOperators &op, int row)
Construct a row operation for a given row.
shape_storage_t::vector_t domain_vector_t
Vector type for vectors in the domain, usually float or double.
int col_offset
Column offset to be used when accessing matrix coefficients.
GradOp(ImplicitVectorOperators &op, int node, int row, const domain_vector_t &vec)
Construct directional derivative operator with given direction.
matrix_t::Scalar scalar_t
Scalar type of matrix elements.
RowVecOp eval(scalar_t alpha) const
Evaluate this operator.
RowVecOp operator-()
Multiply this operation by -1.
Base class for all elementary implicit vector operations.
int row_
Index of the row to which this operation refers.
VecOpBase(ImplicitVectorOperators &op, int node, int row)
Construct operation for a given node.
BasicOp< op_family_t > apply(int node, typename op_family_t::operator_t o, int row)
Add the weights for operator o to the appropriate elements in the matrix.
ImplicitVectorOperators & op
Reference to underlying operators.
ImplicitOperators< shape_storage_t, matrix_t, rhs_t > c(int comp)
Returns ordinary ImplicitOperators for component comp of equation eq_idx.
BasicOp< Lap< dim > > lap(int node)
Same as lap with row equal to current node.
shape_storage_type shape_storage_t
Type of shape storage.
ImplicitVectorOperators & op
Reference to underlying operators.
scalar_t lam
1st Lame constant.
ImplicitVectorOperators()
Default constructor sets offset to 0 and pointers to nullptr.
op_family_t::operator_t o
Basic operator with precomputed shape functions.
This class represents implicit vector operators that fill given matrix M and right hand side rhs with...
BasicOp< op_family_t > apply(int node)
Overload for default-constructible operator.
RowVecOp operator+(const VecOpBase< derived_t > &right)
Add normal operation to a row operation by evaluating the former and adding normally.
Class representing the traction operator. Useful for setting boundary conditions.
int row_offset
Row offset to be used when accessing matrix or rhs coefficients.
RowVecOp eval() const
Eval with alpha = 1.0.
int node
Index of the point for which to apply the operation.
Represents one scalar component of a vector equation.
BasicOp< Lap< dim > > lap(int node, int row)
Sets implicit equation that Laplacian of a vector field is equal to some other value.
ValueOp value(int node)
Same as value with row equal to current node.
void setColOffset(int col_offset)
Sets col offset for given matrix, treating it as is the first column had index col_offset.
Class representing an operation on a specific set of rows of the matrix.