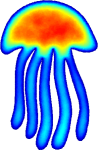 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
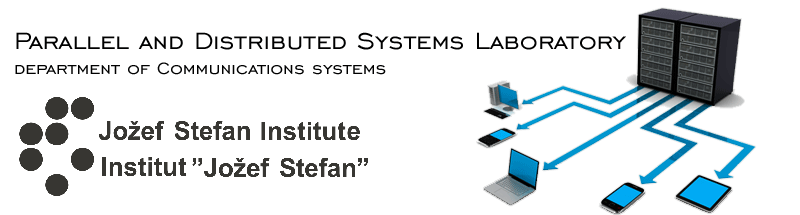 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_EXPLICITVECTOROPERATORS_FWD_HPP_
2 #define MEDUSA_BITS_OPERATORS_EXPLICITVECTOROPERATORS_FWD_HPP_
37 template <
class shape_storage_type>
41 typedef typename shape_storage_t::vector_t
vector_t;
86 template <
class vector_field_t>
88 return apply<Lap<dim>, vector_field_t>(u, node, {});
103 template <
typename op_family_t,
typename vector_field_t>
105 const vector_field_t& u,
int node,
typename op_family_t::operator_t o)
const;
109 template <
typename op_family_t,
typename vector_field_t>
111 return apply<op_family_t, vector_field_t>(u, node, {});
128 template <
class vector_field_t>
131 grad(
const vector_field_t& u,
int node)
const;
142 template <
class vector_field_t>
144 const vector_field_t& u,
int node)
const;
153 template <
class vector_field_t>
169 template <
class vector_field_t>
210 template <
class vector_field_t>
215 template <
typename S>
221 #endif // MEDUSA_BITS_OPERATORS_EXPLICITVECTOROPERATORS_FWD_HPP_
const shape_storage_t * ss
Pointer to shape storage, but name is shortened for readability.
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
void type
By default invalid underlying vector type. Must be specified for each type separately.
shape_storage_t::scalar_t scalar_t
Scalar type.
vector_type< vector_field_t >::type graddiv(const vector_field_t &u, int node) const
Returns gradient of divergence of a vector field u in in node with index node.
@ dim
Number of elements of this matrix.
shape_storage_t::vector_t vector_t
Vector type.
void setShapes(const shape_storage_t &shape_storage_)
Sets a new shape storage from which this operators are generated.
A class for evaluating typical operators needed in spatial discretization.
@ dim
Dimensionality of the function domain.
Type trait for vector fields to obtain their underlying vector type.
friend std::ostream & operator<<(std::ostream &os, const ExplicitVectorOperators< S > &op)
Output basic info about given operators.
ExplicitVectorOperators(const shape_storage_t &ss)
Construct explicit vector operators over given shape storage.
vector_type< vector_field_t >::type curl(const vector_field_t &u, int node) const
Returns curl of a vector field u in node with index node.
vector_type< vector_field_t >::type neumann(const vector_field_t &u, int node, const vector_t &normal, typename vector_type< vector_field_t >::type val) const
This is a vectorised version of ExplicitOperators::neumann.
shape_storage_type shape_storage_t
Type of shape storage.
bool hasShapes() const
Returns true if operators have a non-null pointer to storage and false otherwise.
Eigen::Matrix< typename vector_type< vector_field_t >::type::scalar_t, vector_type< vector_field_t >::type::dim, dim > grad(const vector_field_t &u, int node) const
Returns gradient of vector field u in node with index node.
ExplicitVectorOperators()
Construct empty explicit vector operators.
vector_type< vector_field_t >::type apply(const vector_field_t &u, int node) const
Overload for default constructible operator.
vector_type< vector_field_t >::type::scalar_t div(const vector_field_t &u, int node) const
Returns divergence of a vector field u in in node with index node.
vector_type< vector_field_t >::type lap(const vector_field_t &u, int node) const
Returns Laplacian of vector field u in node with index node.
vector_type< vector_field_t >::type apply(const vector_field_t &u, int node, typename op_family_t::operator_t o) const
Returns an approximation of applying the requested operator to each component of field u at node node...