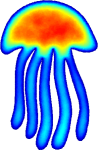 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
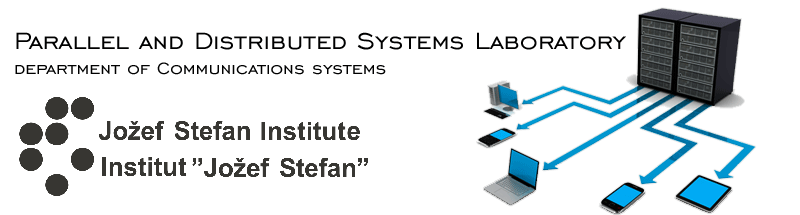 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_EXPLICITOPERATORS_HPP_
2 #define MEDUSA_BITS_OPERATORS_EXPLICITOPERATORS_HPP_
21 #define NODE_ASSERTS(node) \
22 assert_msg(0 <= (node) && (node) < ss->size(), "Node index %d must be in range " \
23 "[0, num_nodes = %d).", ss->size());
26 template <
typename shape_storage_type>
27 template <
typename op_family_t,
typename scalar_field_t>
30 typename op_family_t::operator_t o)
const {
32 int op_idx = op_family_t::index(o);
34 for (
int j = 0; j < ss->supportSize(node); ++j)
35 ret += ss->template get<op_family_t>(op_idx, node, j) * u[ss->support(node, j)];
39 template <
typename shape_storage_type>
40 template <
typename scalar_field_t>
46 for (
int var = 0; var <
dim; ++var) {
47 for (
int i = 0; i < ss->supportSize(node); ++i) {
48 ret[var] += ss->d1(var, node, i) * u[ss->support(node, i)];
55 template <
typename shape_storage_type>
56 template <
typename scalar_field_t>
59 const scalar_field_t& u,
int node,
const vector_t& normal,
62 assert_msg(ss->support(node, 0) == node,
"First support node should be the node itself.");
64 for (
int d = 0; d <
dim; ++d) {
65 for (
int i = 1; i < ss->supportSize(node); ++i) {
66 val -= normal[d] * ss->d1(d, node, i) * u[ss->support(node, i)];
69 denominator += normal[d] * ss->d1(d, node, 0);
72 "Node %d has no effect on the flux in direction %s. The cause of this might be wrong"
73 " normal direction, bad neighbourhood choice or bad nodal positions.", node, normal);
74 return val / denominator;
82 return os <<
"Explicit operators without any linked storage.";
84 return os <<
"Explicit operators over storage: " << *op.
ss;
87 template <
typename Derived,
typename vec_t,
typename OpFamilies>
88 ExplicitOperators<Derived>
95 #endif // MEDUSA_BITS_OPERATORS_EXPLICITOPERATORS_HPP_
const shape_storage_t * ss
Pointer to shape storage, but name is shortened for readability.
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
scalar_type< scalar_field_t >::type neumann(const scalar_field_t &u, int node, const vector_t &normal, typename scalar_type< scalar_field_t >::type val) const
Calculates a new field value a for u at node, such that the Neumann boundary condition du/dn(node) = ...
@ dim
Number of elements of this matrix.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
#define assert_msg(cond,...)
Assert with better error reporting.
#define NODE_ASSERTS(node)
Performs all asserts for a given node: asserts that node index is valid and that shape functions were...
A class for evaluating typical operators needed in spatial discretization.
scalar_type< scalar_field_t >::type apply(const scalar_field_t &u, int node, typename op_family_t::operator_t o) const
Returns an approximation of applying the requested operator to field u at node node.
Vec< typename scalar_type< scalar_field_t >::type, ExplicitOperators< shape_storage_type >::dim > grad(const scalar_field_t &u, int node) const
Returns an approximation of gradient of field u in node-th node.
bool hasShapes() const
Returns true if operators have a non-null pointer to storage and false otherwise.
scalar_field_t::Scalar type
Default scalar type.
@ dim
Dimensionality of the function domain.
ExplicitOperators< Derived > explicitOperators() const
Construct explicit operators over this storage.