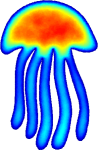 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
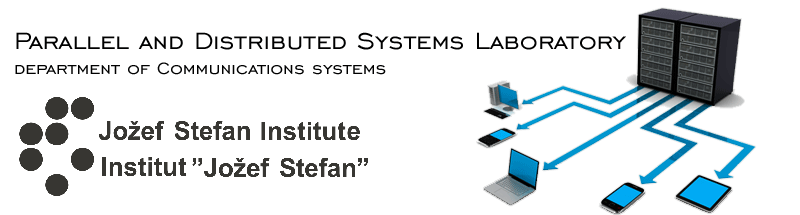 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_INTEGRATORS_ADAMSBASHFORTH_FWD_HPP_
2 #define MEDUSA_BITS_INTEGRATORS_ADAMSBASHFORTH_FWD_HPP_
28 template <
typename Scalar,
int num_steps>
36 Eigen::Matrix<scalar_t, steps, 1>
b_;
45 assert_msg(std::abs(
b_.sum() - 1.0) < 1e-15,
"AB method coefficients do not sum up to 1, "
46 "but %.16f instead.",
b_.sum());
59 template <
typename func_t,
typename initial_method_t = RKExplicit<Scalar, num_steps>>
75 const initial_method_t& initial_method,
86 std::forward_iterator_tag,
94 Eigen::Matrix<scalar_t, Eigen::Dynamic, steps>
last_ys;
136 explicit operator bool() {
149 typename Eigen::Matrix<scalar_t, Eigen::Dynamic, steps>::ConstColXpr
value()
const {
157 typename Eigen::Matrix<scalar_t, Eigen::Dynamic, steps>::ColXpr
value() {
163 return os <<
"t = " <<
step.t <<
"; y = " <<
step.last_ys.col(
steps-1);
185 return os <<
"Multistep integrator using " << integrator.
method_ <<
" method from "
186 <<
"time " << integrator.
t0_ <<
" with step " << integrator.
dt_ <<
" for "
202 template <
typename func_t>
204 const Eigen::VectorXd& y0)
const {
205 return Integrator<func_t, RKExplicit<scalar_t, num_steps>>(
206 *
this, integrators::Explicit::of_order<num_steps>(), func, t0, t_max, dt, y0);
210 template <
typename initial_method_t,
typename func_t>
211 Integrator<func_t, initial_method_t>
solve(
const initial_method_t& method,
const func_t& func,
213 const Eigen::VectorXd& y0)
const {
214 return Integrator<func_t, initial_method_t>(*
this, method, func, t0, t_max, dt, y0);
219 template <
typename func_t>
221 const Eigen::Matrix<scalar_t, Eigen::Dynamic, steps>& last_ys,
223 Eigen::VectorXd result = last_ys.col(
steps-1);
224 for (
int i = 0; i <
steps; ++i) {
225 result += dt *
b_(i) * func(t - i*dt, last_ys.col(i));
232 template <
typename scalar_t,
int stages>
236 namespace integrators {
244 namespace ExplicitMultistep {
247 template <
class scalar_t =
double>
249 Eigen::Matrix<scalar_t, 1, 1> b(1); b << 1.0;
254 template <
class scalar_t =
double>
256 Eigen::Matrix<scalar_t, 2, 1> b(2); b << -1./2., 3./2.;
261 template <
class scalar_t =
double>
263 Eigen::Matrix<scalar_t, 3, 1> b(3); b << 5./12., -4./3., 23./12.;
268 template <
class scalar_t =
double>
270 Eigen::Matrix<scalar_t, 4, 1> b(4); b << -3./8., 37./24., -59./24., 55./24.;
275 template <
class scalar_t =
double>
277 Eigen::Matrix<scalar_t, 5, 1> b(5);
278 b << 251./720., -637./360., 109./30., -1387./360., 1901./720.;
284 namespace of_order_internal {
285 template <
int order,
class scalar_t>
286 struct _of_order_impl {
295 template <
int order,
class scalar_t =
double>
304 #endif // MEDUSA_BITS_INTEGRATORS_ADAMSBASHFORTH_FWD_HPP_
static AdamsBashforth< scalar_t, 2 > AB2()
Two step AB method.
Root namespace for the whole library.
Integrator< func_t > solve(const func_t &func, scalar_t t0, scalar_t t_max, scalar_t dt, const Eigen::VectorXd &y0) const
Returns a solver using this method.
iterator end()
Creates an invalid stepper at positioned past the last step, meant to be used for comparison it == en...
Eigen::VectorXd y0_
initial value
IterationStep & operator*()
Noop, used to conform to std::iterator requirements.
Scalar scalar_t
floating point type
bool is_last() const
Returns true if integrator just completed its last step.
Eigen::Matrix< scalar_t, Eigen::Dynamic, steps >::ConstColXpr value() const
Get current value.
IterationStep(const Integrator &integrator)
Construct an iterator at the initial values of the equation.
Integrator class for AB methods.
static AdamsBashforth< scalar_t, 1 > AB1()
Standard Euler's method.
IterationStep & operator++()
Advance the stepper for one time step, returning the new value.
const func_t & func_
function to integrate
const_iterator cbegin()
Same as Integrator::begin().
Eigen::VectorXd step(const func_t &func, scalar_t t, const Eigen::Matrix< scalar_t, Eigen::Dynamic, steps > &last_ys, scalar_t dt) const
Returns next value.
friend std::ostream & operator<<(std::ostream &os, const AdamsBashforth< scalar_t, stages > &)
Output the method's tableau for debugging.
#define assert_msg(cond,...)
Assert with better error reporting.
IterationStep iterator
iterator type
const initial_method_t initial_method_
method used for first num_steps steps
AdamsBashforth(const Eigen::Matrix< scalar_t, steps, 1 > &b)
Construct an num_steps-step Adams Bashforth method with coefficients b, given as .
static AdamsBashforth< scalar_t, order > of_order()
Returns Adams-Bashforth explicit method of requested order with given floating point type.
IterationStep const_iterator
const iterator type
scalar_t time() const
Get current time.
int iceil(T x)
Ceils a floating point to an integer.
Integrator(const AdamsBashforth< scalar_t, num_steps > &method, const initial_method_t &initial_method, const func_t &func, scalar_t t0, scalar_t t_max, scalar_t dt, Eigen::VectorXd y0)
Constructs stepper with given initial method.
friend std::ostream & operator<<(std::ostream &os, const IterationStep &step)
Output current state of the stepper.
friend std::ostream & operator<<(std::ostream &os, const Integrator &integrator)
Output information about this integrator.
const_iterator cend()
Same as Integrator::end().
Eigen::Matrix< scalar_t, steps, 1 > b_
coefficients of the multistep method
static AdamsBashforth< scalar_t, 4 > AB4()
Four step AB method.
iterator begin()
Creates stepper at positioned at initial value.
int max_steps
number of steps
bool operator!=(const IterationStep &other) const
Negation of IterationStep::operator==.
const IterationStep & operator*() const
const version of IterationStep::operator*
IterationStep operator++(int)
Advance the stepper for one time step, returning the old value.
static AdamsBashforth< scalar_t, 5 > AB5()
Five step AB method.
bool operator==(const IterationStep &other) const
Compare two steppers if they are on the same step.
@ steps
number of steps of the multistep method
const AdamsBashforth< scalar_t, num_steps > method_
method used for all next steps
static AdamsBashforth< scalar_t, 3 > AB3()
Three step AB method.
scalar_t & time()
Read-write access to current time.
Class representing an AdamsBashforth method, an explicit linear multistep method.
Eigen::Matrix< scalar_t, Eigen::Dynamic, steps >::ColXpr value()
Read-write access to current value.
const Integrator & integrator
reference to underlying integrator
Integrator< func_t, initial_method_t > solve(const initial_method_t &method, const func_t &func, scalar_t t0, scalar_t t_max, scalar_t dt, const Eigen::VectorXd &y0) const
Same as AdamsBashforth::solve, but offers the option of supplying your own initial method.
Class representing a step in the integration process.
IterationStep(const Integrator &integrator, int)
Construct an (invalid) iterator pointing past the last step.
Eigen::Matrix< scalar_t, Eigen::Dynamic, steps > last_ys
current value