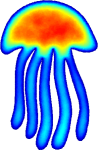 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
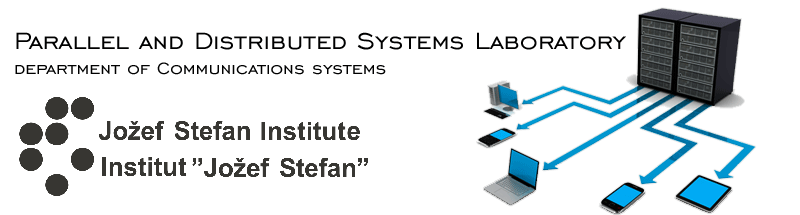 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_UTILS_ASSERT_HPP_
2 #define MEDUSA_BITS_UTILS_ASSERT_HPP_
9 #include <tinyformat/tinyformat.h>
16 #define VA_NUM_ARGS(...) VA_NUM_ARGS_IMPL(__VA_ARGS__, 5, 4, 3, 2, 1, 0)
17 #define VA_NUM_ARGS_IMPL(_1, _2, _3, _4, _5, N, ...) N
18 #define macro_dispatcher(func, ...) macro_dispatcher_(func, VA_NUM_ARGS(__VA_ARGS__))
19 #define macro_dispatcher_(func, nargs) macro_dispatcher__(func, nargs)
20 #define macro_dispatcher__(func, nargs) func ## nargs
21 #define addflag(a) {std::cerr << "flags=[flags, " << (a) << "];" << std::endl;}
22 #define prnv2(a, b) {std::cerr << a << " = " << (b) << ";" << std::endl;}
23 #define prnv1(a) {std::cerr << #a << " = " << (a) << ";" << std::endl;}
34 #define prn(...) macro_dispatcher(prnv, __VA_ARGS__)(__VA_ARGS__)
36 using tinyformat::printf;
37 using tinyformat::format;
40 namespace assert_internal {
51 int line,
const char* message, tfm::FormatListRef format_list);
53 template<
typename... Args>
54 bool assert_handler(
const char* condition,
const char* file,
const char* func_name,
int line,
55 const char* message,
const Args&... args) {
56 tfm::FormatListRef arg_list = tfm::makeFormatList(args...);
62 #define assert_msg(cond, ...) ((void)sizeof(cond))
75 #define assert_msg(cond, ...) ((void)(!(cond) && \
76 mm::assert_internal::assert_handler( \
77 #cond, __FILE__, __PRETTY_FUNCTION__, __LINE__, __VA_ARGS__) && (assert(0), 1)))
85 inline void print_red(
const std::string& s) { std::cout <<
"\x1b[31;1m" << s <<
"\x1b[37;0m"; }
90 inline void print_white(
const std::string& s) { std::cout <<
"\x1b[37;1m" << s <<
"\x1b[37;0m"; }
95 inline void print_green(
const std::string& s) { std::cout <<
"\x1b[32;1m" << s <<
"\x1b[37;0m"; }
99 #endif // MEDUSA_BITS_UTILS_ASSERT_HPP_
Root namespace for the whole library.
bool assert_handler_implementation(const char *condition, const char *file, const char *func_name, int line, const char *message, tfm::FormatListRef format_list)
Actual assert implementation.
void print_green(const std::string &s)
Prints given text in bold green.
bool assert_handler(const char *condition, const char *file, const char *func_name, int line, const char *message, const Args &... args)
Assert handler that unpacks varargs.
void print_red(const std::string &s)
Prints given text in bold red.
void print_white(const std::string &s)
Prints given text in bold white.