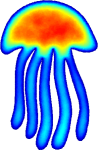 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
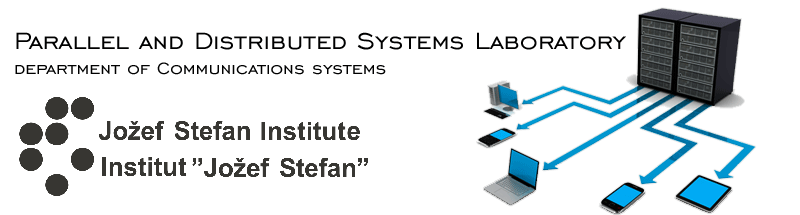 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_SPATIAL_SEARCH_KDGRID_HPP_
2 #define MEDUSA_BITS_SPATIAL_SEARCH_KDGRID_HPP_
17 template <
typename vec_t>
21 for (
int i = 0; i <
dim; ++i) {
22 assert_msg(bot[i] <= top[i],
"Bottom bounds %s must be below top bounds %s.", bot, top);
23 counts[i] =
iceil((top[i] - bot[i]) / cell_size) + 1;
28 template <
typename vec_t>
30 for (
int i = 0; i <
dim; ++i) {
31 if (cur[i] == ref[i] + span) {
32 cur[i] = ref[i] - span;
41 template <
typename vec_t>
43 assert_msg(grid_(index) == -1,
"This grid cell is already occupied by point %d. Consider"
44 " using smaller cell size.", grid_(index));
45 int idx = points_.size();
51 template <
typename vec_t>
54 int span =
static_cast<int>(std::ceil(r/cell_size_));
57 for (
int i = 0; i <
dim; ++i) tmp_index[i] -= span;
59 if (grid_.inBounds(tmp_index) && grid_(tmp_index) != -1) {
60 auto gp = points_[grid_(tmp_index)];
61 if ((p-gp).squaredNorm() < r2) {
65 }
while (increment(tmp_index, span, index));
69 template <
typename vec_t>
71 Eigen::Matrix<scalar_t, dim, 1> idx = (p-bot_) / cell_size_;
73 for (
int i = 0; i <
dim; ++i) {
74 index[i] =
static_cast<int>(idx[i]);
82 os <<
"KDGrid search structure from " << search.
bot_ <<
" to " << search.
top_
83 <<
" with cell_size " << search.
cell_size_ <<
" containing " << search.
size()
91 #endif // MEDUSA_BITS_SPATIAL_SEARCH_KDGRID_HPP_
Root namespace for the whole library.
int size() const
Get number of points stored in the structure.
bool existsPointInSphere(const vec_t &p, scalar_t r)
Check if any point exists in sphere centered at p with radius r.
@ dim
Number of elements of this matrix.
IndexArray compute_index(const vec_t &p)
Compute point multi-index.
vec_t top_
Upper bound of the box.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
#define assert_msg(cond,...)
Assert with better error reporting.
Grid< int, dim >::IndexArray IndexArray
Multi-index type.
int iceil(T x)
Ceils a floating point to an integer.
vec_t bot_
Lower bound of the box.
Search structure over given d-dimensional box with given cell size.
static IndexArray compute_size(const vec_t &bot, const vec_t &top, scalar_t cell_size)
Compute the sizes of the rid along each dimension.
vector_t::scalar_t scalar_t
Scalar type used.
scalar_t cell_size_
Size of a single cell.
int insert(const vec_t &p)
Insert a new point in the structure.
bool increment(IndexArray &cur, int span, const IndexArray &ref)
Increment a dim-ary counter.