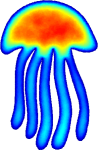 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
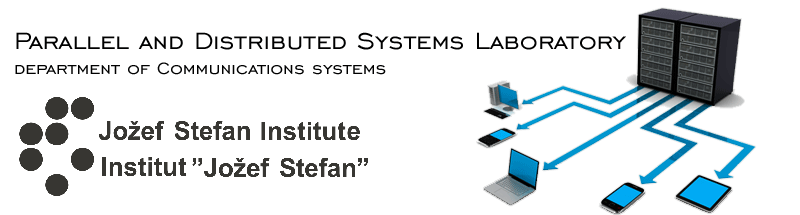 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_SPATIAL_SEARCH_KDGRID_FWD_HPP_
2 #define MEDUSA_BITS_SPATIAL_SEARCH_KDGRID_FWD_HPP_
28 template <
typename vec_t>
76 void insert(
const std::vector<vec_t>& pts) {
77 for (
const vec_t& p : pts)
insert(p);
108 #endif // MEDUSA_BITS_SPATIAL_SEARCH_KDGRID_FWD_HPP_
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
const Grid< int, dim > & grid() const
Get access to underlying grid of point indices.
int size() const
Get number of points stored in the structure.
const vec_t & bottom() const
Get bottom bound.
bool existsPointInSphere(const vec_t &p, scalar_t r)
Check if any point exists in sphere centered at p with radius r.
@ dim
Number of elements of this matrix.
IndexArray compute_index(const vec_t &p)
Compute point multi-index.
vec_t top_
Upper bound of the box.
@ dim
Dimensionality of the space.
void insert(const std::vector< vec_t > &pts)
Vectorised version of insert.
Grid< int, dim >::IndexArray IndexArray
Multi-index type.
const vec_t & top() const
Get top bound.
const std::vector< vec_t > & points() const
Get access to array of stored points.
vec_t bot_
Lower bound of the box.
vec_t vector_t
Vector type used.
const vec_t & point(int idx) const
Get point by its sequential index.
Search structure over given d-dimensional box with given cell size.
scalar_t cellSize() const
Get cell size.
friend std::ostream & operator<<(std::ostream &os, const KDGrid< V > &search)
Output some information about the search grid.
static IndexArray compute_size(const vec_t &bot, const vec_t &top, scalar_t cell_size)
Compute the sizes of the rid along each dimension.
vector_t::scalar_t scalar_t
Scalar type used.
scalar_t cell_size_
Size of a single cell.
KDGrid(const vec_t &bot, const vec_t &top, scalar_t cell_size)
Construct a search grid from bot to top with given cell_size.
int insert(const vec_t &p)
Insert a new point in the structure.
std::vector< vec_t > points_
List of inserted points.
Grid< int, dim > grid_
Background grid of cells pointing to sequential point indices.
bool increment(IndexArray &cur, int span, const IndexArray &ref)
Increment a dim-ary counter.