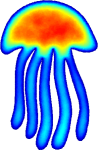 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
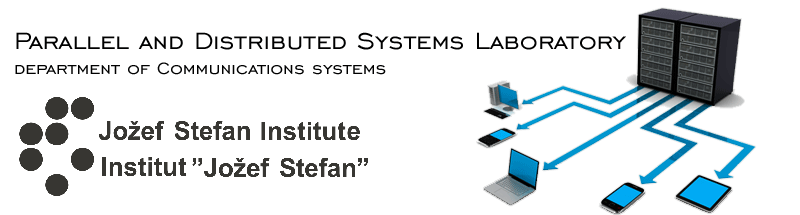 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_IMPLICITVECTOROPERATORS_HPP_
2 #define MEDUSA_BITS_OPERATORS_IMPLICITVECTOROPERATORS_HPP_
15 template <
class shape_storage_type,
class matrix_type,
class rhs_type>
18 ss(&ss), M(&M), rhs(&rhs), row_offset(row_offset), col_offset(col_offset) {
22 "Matrix does not have enough rows, expected at least %d = %d + %d, got %d.",
25 "Matrix does not have enough columns, expected at least %d = %d + %d, got %d.",
28 "RHS vector does not have enough rows, expected at least %d = %d + %d, got %d.",
32 if (
M.squaredNorm() > 1e-10) {
33 std::cerr <<
"Warning: matrix in implicit operators not initialized to zero!" << std::endl;
35 if (
rhs.squaredNorm() > 1e-10) {
36 std::cerr <<
"Warning: rhs in implicit operators not initialized to zero!" << std::endl;
42 template <
typename S,
typename M,
typename R>
44 os <<
"Implicit vector operators ";
45 os << ((op.
hasShapes()) ?
"with" :
"without") <<
" linked storage ";
47 os <<
"without linked storage";
49 os <<
"over storage: " << *op.
ss;
51 os <<
" with " << ((op.
hasMatrix()) ?
"defined" :
"undefined") <<
" problem matrix";
52 os <<
" and with " << ((op.
hasRhs()) ?
"defined" :
"undefined") <<
" problem rhs.";
57 template <
typename Derived,
typename vec_t,
typename OpFamilies>
58 template <
typename M,
typename R>
59 ImplicitVectorOperators<Derived, M, R>
61 return {*
static_cast<const Derived*
>(
this), matrix, rhs};
66 #endif // MEDUSA_BITS_OPERATORS_IMPLICITVECTOROPERATORS_HPP_
bool hasMatrix() const
Returns true if operators have a non-null pointer to problem matrix.
rhs_type rhs_t
Right hand side type.
Root namespace for the whole library.
bool hasRhs() const
Returns true if operators have a non-null pointer to problem right hand side.
rhs_t * rhs
Pointer to right hand side.
matrix_type matrix_t
Matrix type.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
#define assert_msg(cond,...)
Assert with better error reporting.
matrix_t * M
Pointer to problem matrix.
@ dim
Dimensionality of the domain.
bool hasShapes() const
Returns true if operators have a non-null pointer to storage and false otherwise.
const shape_storage_t * ss
Shape storage, but name is shortened for readability.
int col_offset
Column offset to be used when accessing matrix coefficients.
ImplicitVectorOperators< Derived, M, R > implicitVectorOperators(M &matrix, R &rhs) const
Construct implicit vector operators over this storage.
shape_storage_type shape_storage_t
Type of shape storage.
ImplicitVectorOperators()
Default constructor sets offset to 0 and pointers to nullptr.
This class represents implicit vector operators that fill given matrix M and right hand side rhs with...
int row_offset
Row offset to be used when accessing matrix or rhs coefficients.