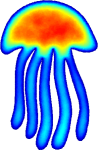 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
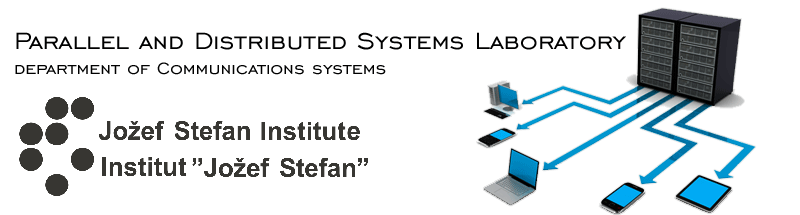 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_GRIDFILL_HPP_
2 #define MEDUSA_BITS_DOMAINS_GRIDFILL_HPP_
17 template <
typename vec_t>
19 for (
int i = 0; i <
dim; ++i) {
24 template <
typename vec_t>
27 if (type == 0) type = 1;
29 for (
int i = 0; i <
dim; ++i) {
30 counts[i] =
iceil((top[i] - bot[i]) / h) + 1;
34 for (
const auto& x :
linspace(bot, top, counts)) {
35 if (domain.
contains(x) && (tree.
size() == 0 || tree.
query(x, 1).second[0] >= h*h)) {
43 #endif // MEDUSA_BITS_DOMAINS_GRIDFILL_HPP_
Root namespace for the whole library.
vec_t bot
Bottom left corner of the box to fill.
Class representing domain discretization along with an associated shape.
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
Class representing a static k-d tree data structure.
@ dim
Dimensionality of the domain.
vec_t::scalar_t scalar_t
Scalar type;.
@ dim
Number of elements of this matrix.
GridFill(const vec_t &bot, const vec_t &top)
Prepare to fill domain within [bot, top] bounding box.
const Range< vec_t > & positions() const
Returns positions of all nodes.
#define assert_msg(cond,...)
Assert with better error reporting.
std::pair< Range< int >, Range< scalar_t > > query(const vec_t &point, int k=1) const
Find k nearest neighbors to given point.
int iceil(T x)
Ceils a floating point to an integer.
Range< Vec< scalar_t, dim > > linspace(const Vec< scalar_t, dim > &beg, const Vec< scalar_t, dim > &end, const Vec< int, dim > &counts, const Vec< bool, dim > include_boundary=true)
Multidimensional clone of Matlab's linspace function.
bool contains(const vec_t &point) const
Returns true if point is inside the domain.
int size() const
Returns number of points in this tree.
vec_t top
Top right corner of the box to fill.
int addInternalNode(const vec_t &point, int type)
Adds a single interior node with specified type to this discretization.
void operator()(DomainDiscretization< vec_t > &domain, const scalar_t &h, int type=0) const
Fills domain with a grid node distribution with spacing h.