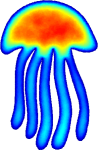 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
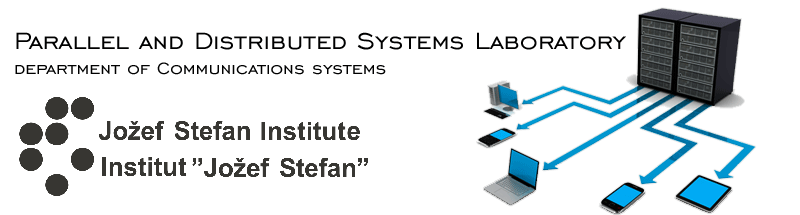 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_DOMAINDISCRETIZATION_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_DOMAINDISCRETIZATION_FWD_HPP_
21 template <
typename vec_t,
typename OpFamilies>
45 template <
class vec_t>
123 template <
typename hdf5_file>
177 const vec_t&
normal(
int i)
const;
241 template <
typename func_t>
275 template <
typename func_t>
300 template <
typename compare_t = std::less<std::pair<vec_t,
int>>>
399 bool contains(
const vec_t& point)
const;
415 template <
typename search_structure_t>
416 bool discreteContains(
const vec_t& point,
const search_structure_t& search)
const;
424 template <
typename search_structure_t>
425 bool contains(
const vec_t& point,
const search_structure_t& search)
const;
433 template <
typename search_structure_t>
441 #define DOMAIN_PLUGIN(Name) \
442 template<typename callable_t, typename... Args> \
443 void Name(callable_t& callable, Args&&... args) { \
444 return callable(*this, std::forward<Args>(args)...); \
448 #define DOMAIN_PLUGIN_CONST(Name) \
449 template<typename callable_t, typename... Args> \
450 void Name(const callable_t& callable, Args&&... args) { \
451 callable(*this, std::forward<Args>(args)...); \
472 approx_t approx, const
indexes_t& indexes = {})
const;
474 template <
typename OperatorTuple,
typename approx_t>
476 approx_t approx,
const indexes_t& indexes = {})
const;
478 template <
typename OperatorTuple,
typename approx_t>
479 RaggedShapeStorage<vec_t, OperatorTuple>
computeShapes(OperatorTuple operators,
480 approx_t approx,
const indexes_t& indexes = {})
const;
483 friend std::ostream&
operator<<(std::ostream& os,
const DomainDiscretization<V>& d);
486 std::ostream&
output_report(std::ostream& os = std::cout)
const;
490 template <
class vec_t>
498 #endif // MEDUSA_BITS_DOMAINS_DOMAINDISCRETIZATION_FWD_HPP_
Range< int > boundary_map_
Mapping index of a boundary node among all nodes, to its index among boundary nodes.
int support(int i, int j) const
Returns j-th support node of i-th node.
Root namespace for the whole library.
indexes_t addNodes(const DomainDiscretization< vec_t > &d)
Add nodes from another discretization to this discretization.
scalar_t dr(int i) const
Returns Euclidean distance to the second support node.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
indexes_t interior() const
Returns indexes of all internal nodes.
DomainDiscretization & add(const DomainDiscretization &d)
Merges given discretization to the current discretization.
Range< int > reorderNodes(const compare_t &cmp=compare_t())
Reorders nodes according to the compare function.
indexes_t all() const
Returns indexes of all nodes, i.e. {0, 1, ..., N-1}.
void chopOff(const DomainDiscretization< vec_t > &d, const Range< int > &relevant)
Remove a portion of this domain which is contained in d or is only dx away.
void removeInternalNodes()
Removes all internal nodes.
vec_t & pos(int i)
Returns writeable position of i-th node.
const Range< int > & bmap() const
Returns boundary map.
void clear()
Clears all data about this discretization.
Range< int > types_
Storing types of nodes aligned with positions.
const Range< vec_t > & normals() const
Returns normals of all boundary nodes.
DomainDiscretization & translate(const vec_t &a)
Translate the domain by a given vector a.
std::ostream & output_report(std::ostream &os=std::cout) const
Outputs a simple report about out domain, like number of nodes, support size.
@ dim
Number of elements of this matrix.
friend std::ostream & operator<<(std::ostream &os, const DomainDiscretization< V > &d)
Output basic info about given domain.
unsigned int shape_flags
Type representing flags for shape functions.
Range< vec_t > supportNodes(int i) const
Returns positions of support nodes of i-th node.
void assert_is_valid() const
Checks if domain is in valid state.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
const Range< vec_t > & positions() const
Returns positions of all nodes.
DomainDiscretization & operator-=(const DomainDiscretization &d)
Operator version of DomainDiscretization::subtract.
Range< Range< int > > support_
Attribute used to store support points of each node.
@ dim
Dimensionality of the domain.
Range< int > addGhostNodes(scalar_t h, int type=0)
Overload of addGhostNodes with constant h and adds ghost nodes to all boundary nodes.
Base class for geometric shapes of domains.
int supportSize(int i) const
Returns size of i-th node support.
const Range< int > & types() const
Returns types of all nodes.
#define DOMAIN_PLUGIN_CONST(Name)
Const version of DOMAIN_PLUGIN.
const vec_t & normal(int i) const
Returns outside unit normal of i-th node.
void changeToBoundary(int i, const vec_t &point, int type, const vec_t &normal)
Changes node i to boundary point with given type and normal.
#define DOMAIN_PLUGIN(Name)
Define a method Name that calls its first argument.
int size() const
Returns N, the number of nodes in this discretization.
const DomainShape< vec_t > & shape() const
Returns geometric shape of the underlying domain.
int type(int i) const
Returns type of i-th node.
void relax(callable_t &callable, Args &&... args)
Enables more readable calls to relax engines.
std::vector< int > indexes_t
Class representing a collection of indices.
int & type(int i)
Returns writeable type of i-th node.
bool contains(const vec_t &point) const
Returns true if point is inside the domain.
void makeDiscreteContainsStructure(search_structure_t &search) const
Fills the search structure search with boundary points for use with contains() or discreteContains().
void findSupport(callable_t &callable, Args &&... args)
Enables more readable calls to support engines.
int bmap(int node) const
Returns index of node node among only boundary nodes.
bool discreteContains(const vec_t &point, const search_structure_t &search) const
A discrete version of contains using given boundary discretization.
DomainDiscretization & rotate(const Eigen::Matrix< scalar_t, dim, dim > &Q)
Transform the domain by given orthogonal matrix Q.
Range< int > & support(int i)
Returns writeable array of support indices for i-th node.
void fill(callable_t &callable, Args &&... args)
Enables more readable calls to fill engines.
void removeNodes(const Range< int > &to_remove)
Remove nodes with given indices. This removes their types and potential normals as well.
vec_t vector_t
Vector data type used in computations.
int addInternalNode(const vec_t &point, int type)
Adds a single interior node with specified type to this discretization.
const vec_t & pos(int i) const
Returns the position of i-th node.
DomainDiscretization & subtract(const DomainDiscretization &d)
Subtracts given discretized domain from *this.
Efficiently stores shape functions of different lengths.
indexes_t boundary() const
Returns indexes of all boundary nodes.
vec_t supportNode(int i, int j) const
Returns position of j-th support node of i-th node.
void removeBoundaryNodes()
Removes all boundary nodes.
int size() const
Returns number of elements.
RaggedShapeStorage< vec_t, typename sh::operator_tuple< mask, vec_t::dim >::type > computeShapes(approx_t approx, const indexes_t &indexes={}) const
Compute shapes, specified with shape flags, for this domain with given approximation for given indexe...
void shuffleNodes(const indexes_t &permutation)
Shuffles node order according to given permutation.
static DomainDiscretization< vec_t > load(hdf5_file &file, const std::string &name)
Load discretization from a HD5 file.
vec_t::Scalar scalar_t
Scalar data type used in computation.
Range< int > supportSizes() const
Returns a vector of support sizes for each node.
DomainDiscretization & operator+=(const DomainDiscretization &d)
Operator version of DomainDiscretization::add.
const Range< int > & support(int i) const
Returns support indices for i-th node.
DomainDiscretization(const DomainShape< vec_t > &shape)
Construct an empty discretization for a given shape.
Range< int > & types()
Returns mutable types of all nodes.
Range< vec_t > normals_
List of normals of boundary nodes.
Range< vec_t > positions_
Positions of internal discretization points.
scalar_t pos(int i, int j) const
Returns j-th coordinate of the position of i-th node.
void changeToInterior(int i, const vec_t &point, int type)
Changes node i to interior point with given type.
const Range< Range< int > > & supports() const
Returns support indices for all nodes.
int addNode(const vec_t &point, int type)
Helper for adding points to the domain, which keeps the object consistent.
deep_copy_unique_ptr< DomainShape< vec_t > > shape_
Geometric shape of the domain.
Unique pointer with polymorphic deep copy semantics.
int addBoundaryNode(const vec_t &point, int type, const vec_t &normal)
Adds a boundary node with given type and normal to the domain.