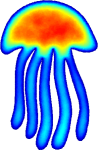 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
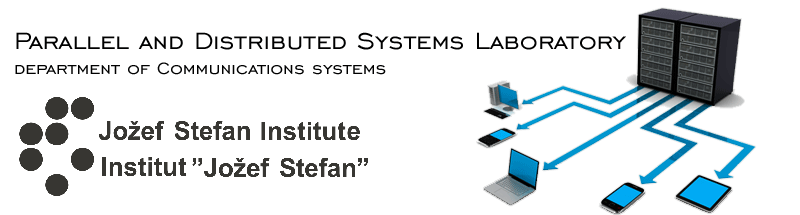 |
Go to the documentation of this file.
14 "Label '%s' already exists. Use stopwatch to time repeatedly.", label);
16 times_.push_back(std::chrono::steady_clock::now());
20 os <<
"Not enough checkpoints.";
24 for (
const auto& label :
labels_) M = std::max(M, label.size());
25 for (
size_t c = 1; c <
labels_.size(); ++c) {
27 << std::setw(M) <<
labels_[c - 1] <<
" -- " << std::setw(M) <<
labels_[c]
28 <<
' ' << std::setw(10) << std::scientific
29 << std::chrono::duration<double>(
times_[c] -
times_[c-1]).count() <<
" [s]"
32 os << std::left << std::setw(2*M+5)
33 <<
"total time " << std::setw(10)
34 << std::chrono::duration<double>(
times_.back() -
times_[0]).count()
35 <<
" [s]" << std::endl;
39 std::ostream& os)
const {
44 assert_msg(0 <= from && from <
size(),
"From ID %d out of range [0, %d).", from,
size());
46 os << std::left << std::setw(20) <<
labels_[from] <<
" -- "
47 << std::setw(20) <<
labels_[to] << std::setw(18)
48 << std::chrono::duration<double>(
times_[to] -
times_[from]).count()
49 <<
"[s]" << std::endl;
64 return std::chrono::duration<double>(
65 std::chrono::steady_clock::now() -
times_[
getID(from)]).count();
Root namespace for the whole library.
double durationToNow(const std::string &from) const
Return time difference in seconds between from and now.
int getID(const std::string &label) const
Returns the ID of checkpoints with a given label.
#define assert_msg(cond,...)
Assert with better error reporting.
void addCheckPoint(const std::string &label)
Adds a checkpoint with given label and remembers the time at which it was added.
std::chrono::steady_clock::time_point time_type
Time type.
time_type timeAt(int id) const
Return absolute time for a given id.
std::vector< time_type > times_
List of checkpoint times.
std::vector< std::string > labels_
List of checkpoint labels.
int size() const
Return the number of measurements taken.
void showTimings(int from, int to, std::ostream &os=std::cout) const
Output timings between the checkpoints with given ids to os.
void clear()
Clear all of time points.
double duration(const std::string &from, const std::string &to) const
Return time difference in seconds between two checkpoints.