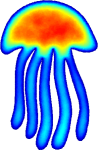 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
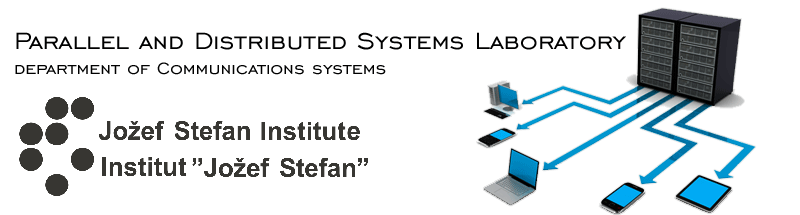 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_UTILS_TIMER_HPP_
2 #define MEDUSA_BITS_UTILS_TIMER_HPP_
31 typedef std::chrono::steady_clock::time_point
time_type;
36 void showTimings(
int from,
int to, std::ostream& os = std::cout)
const;
38 int getID(
const std::string& label)
const;
49 void showTimings(std::ostream& os = std::cout)
const;
54 void showTimings(
const std::string& from,
const std::string& to,
55 std::ostream& os = std::cout)
const;
59 double duration(
const std::string& from,
const std::string& to)
const;
67 const std::vector<time_type>&
times()
const {
return times_; }
80 #endif // MEDUSA_BITS_UTILS_TIMER_HPP_
Root namespace for the whole library.
double durationToNow(const std::string &from) const
Return time difference in seconds between from and now.
friend std::ostream & operator<<(std::ostream &os, const Timer &timer)
Output all times between all checkpoints.
int getID(const std::string &label) const
Returns the ID of checkpoints with a given label.
void addCheckPoint(const std::string &label)
Adds a checkpoint with given label and remembers the time at which it was added.
std::chrono::steady_clock::time_point time_type
Time type.
Simple timer class: add checkpoints throughout the code and measure execution time between them.
time_type timeAt(int id) const
Return absolute time for a given id.
const std::vector< std::string > & labels() const
Return all labels.
std::vector< time_type > times_
List of checkpoint times.
const std::vector< time_type > & times() const
Return all times.
std::vector< std::string > labels_
List of checkpoint labels.
int size() const
Return the number of measurements taken.
void showTimings(int from, int to, std::ostream &os=std::cout) const
Output timings between the checkpoints with given ids to os.
void clear()
Clear all of time points.
double duration(const std::string &from, const std::string &to) const
Return time difference in seconds between two checkpoints.