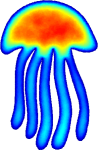 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
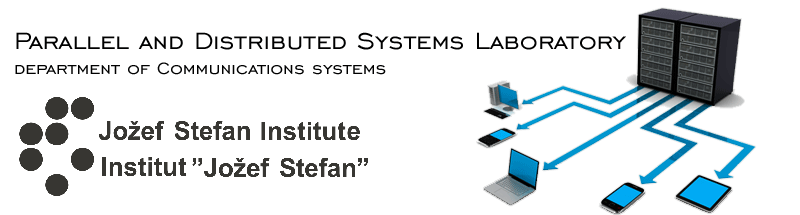 |
Go to the documentation of this file.
14 auto it = std::find(
labels.begin(),
labels.end(), label);
15 assert_msg(it !=
labels.end(),
"Label '%s' not found. Available labels: %s.",
17 return it -
labels.begin();
20 for (
int i = 0; i < static_cast<int>(
labels.size()); i++) {
23 "start() for label '%s' was called more than once"
24 " before stop() call.", label.c_str());
25 times[i] = std::chrono::steady_clock::now();
32 times.push_back(std::chrono::steady_clock::now());
38 int id =
getID(label);
40 "stop() for label '%s' was called"
41 " more than once before start() call.",
45 std::chrono::steady_clock::now()
51 "Stopwatch with label '%s' is still running, `stop()` must be called"
52 " before results can be displayed.", label.c_str());
57 "Stopwatch with label '%s' is still running, `stop()` must be called"
58 " before results can be displayed.", label.c_str());
63 "Stopwatch with label '%s' is still running, `stop()` must be called"
64 " before results can be displayed.", label.c_str());
78 for (
const auto& label : stopwatch.
labels) {
79 if (label.size() > maxs) {
83 for (
const auto& label : stopwatch.
labels) {
84 os << std::setw(maxs) << label <<
": " << stopwatch.
timePerLap(label) <<
" [s]\n";
std::vector< std::string > labels
List of stopwatch labels.
Root namespace for the whole library.
int numLaps(const std::string &label) const
Returns number of laps for a given label.
void clear()
Clear all stopwatch related data.
std::vector< double > cumulative_time
List of cumulative times for each stopwatch.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
#define assert_msg(cond,...)
Assert with better error reporting.
double cumulativeTime(const std::string &label) const
Returns total time of all laps for a given label.
A simple stopwatch class: time sections of code that execute repeatedly and get average execution tim...
double timePerLap(const std::string &label) const
Returns average time spent per lap.
std::vector< bool > currently_running
For tracking when stopwatch is running.
void start(const std::string &label)
Starts the stopwatch with a given label.
std::vector< time_type > times
List of times for each stopwatch.
bool isRunning(const std::string &label) const
Returns if stopwatch with a given label is currently running.
std::vector< int > counts
List of lap counts for each stopwatch.
void stop(const std::string &label)
Stops the stopwatch with a given label.
int getID(const std::string &label) const
Returns the ID of stopwatch with a given label.