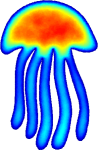 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
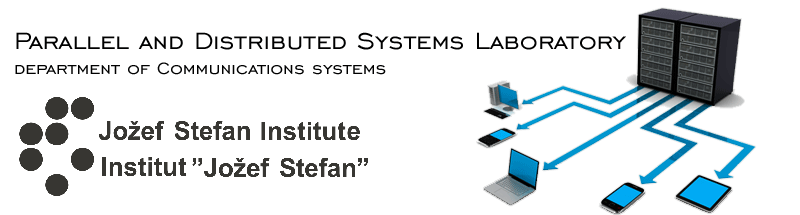 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_UTILS_STOPWATCH_HPP_
2 #define MEDUSA_BITS_UTILS_STOPWATCH_HPP_
32 typedef std::chrono::steady_clock::time_point
time_type;
40 int getID(
const std::string& label)
const;
44 void start(
const std::string& label);
50 void stop(
const std::string& label);
54 int numLaps(
const std::string& label)
const;
56 double timePerLap(
const std::string& label)
const;
67 #endif // MEDUSA_BITS_UTILS_STOPWATCH_HPP_
std::vector< std::string > labels
List of stopwatch labels.
Root namespace for the whole library.
int numLaps(const std::string &label) const
Returns number of laps for a given label.
void clear()
Clear all stopwatch related data.
std::vector< double > cumulative_time
List of cumulative times for each stopwatch.
double cumulativeTime(const std::string &label) const
Returns total time of all laps for a given label.
A simple stopwatch class: time sections of code that execute repeatedly and get average execution tim...
double timePerLap(const std::string &label) const
Returns average time spent per lap.
std::chrono::steady_clock::time_point time_type
Time type.
std::vector< bool > currently_running
For tracking when stopwatch is running.
void start(const std::string &label)
Starts the stopwatch with a given label.
std::vector< time_type > times
List of times for each stopwatch.
bool isRunning(const std::string &label) const
Returns if stopwatch with a given label is currently running.
friend std::ostream & operator<<(std::ostream &os, const Stopwatch &stopwatch)
Output average lap times for all labels.
std::vector< int > counts
List of lap counts for each stopwatch.
void stop(const std::string &label)
Stops the stopwatch with a given label.
int getID(const std::string &label) const
Returns the ID of stopwatch with a given label.