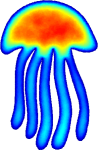 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
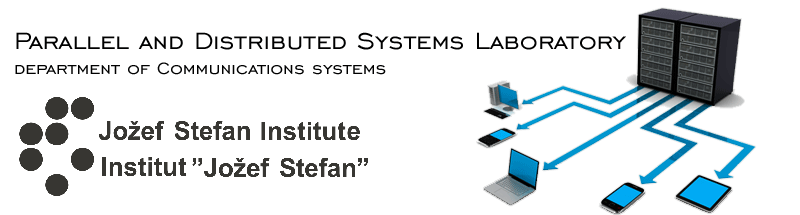 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_SHAPEDIFFERENCE_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_SHAPEDIFFERENCE_FWD_HPP_
25 template <
typename vec_t>
41 sh2->setMargin(-
sh2->margin());
49 bool contains(
const vec_t& point)
const override {
50 return sh1->contains(point) && !
sh2->contains(point);
54 return sh1->hasContains() &&
sh2->hasContains();
59 scalar_t step,
int internal_type,
int boundary_type)
const override;
61 const std::function<
scalar_t(vec_t)>& dr,
int type)
const override;
63 const std::function<
scalar_t(vec_t)>& dr,
int internal_type,
64 int boundary_type)
const override;
66 std::pair<vec_t, vec_t>
bbox()
const override {
return sh1->bbox(); }
68 std::ostream&
print(std::ostream& os)
const override;
73 #endif // MEDUSA_BITS_DOMAINS_SHAPEDIFFERENCE_FWD_HPP_
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
Base class for geometric shapes of domains.
const DomainShape< vec_t > & second() const
Access the second shape.
bool hasContains() const override
Return true if shape has contains() method implemented.
DomainDiscretization< vec_t > discretizeWithStep(scalar_t step, int internal_type, int boundary_type) const override
Returns a discretization of this shape with approximately uniform distance step between nodes.
ShapeDifference(const DomainShape< vec_t > &shape1, const DomainShape< vec_t > &shape2)
Constructs a shape representing shape1 - shape2.
ShapeDifference< vec_t > * clone() const override
Polymorphic clone pattern.
deep_copy_unique_ptr< DomainShape< vec_t > > sh2
Second shape.
std::ostream & print(std::ostream &os) const override
Output information about this shape to given output stream os.
std::pair< vec_t, vec_t > bbox() const override
Return the bounding box of the domain.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const override
Discretizes boundary with given density and fill engine.
A class representing a set-difference of two shapes.
DomainDiscretization< vec_t > discretizeWithDensity(const std::function< scalar_t(vec_t)> &dr, int internal_type, int boundary_type) const override
Returns a discretization of the domain with spatially variable step.
bool contains(const vec_t &point) const override
Return true if point is not more than margin() outside the domain.
DomainDiscretization< vec_t > discretizeBoundaryWithStep(scalar_t step, int type) const override
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
Unique pointer with polymorphic deep copy semantics.
vec_t::Scalar scalar_t
Scalar data type used in computation.
const DomainShape< vec_t > & first() const
Access the first shape.
deep_copy_unique_ptr< DomainShape< vec_t > > sh1
First shape.