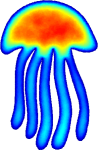 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
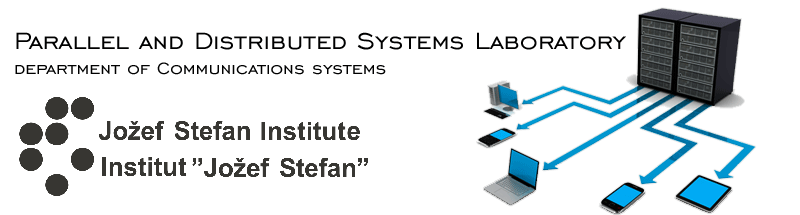 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_SHAPEDIFFERENCE_HPP_
2 #define MEDUSA_BITS_DOMAINS_SHAPEDIFFERENCE_HPP_
16 template <
typename vec_t>
19 auto d1 = sh1->discretizeBoundaryWithStep(step, type);
21 auto d2 = sh2->discretizeBoundaryWithStep(step, type);
23 return d1.subtract(
d2);
26 template <
typename vec_t>
28 scalar_t step,
int internal_type,
int boundary_type)
const {
29 auto d1 = sh1->discretizeWithStep(step, internal_type, boundary_type);
31 auto d2 = sh2->discretizeBoundaryWithStep(step, boundary_type);
33 return d1.subtract(
d2);
36 template <
typename vec_t>
38 const std::function<
scalar_t(vec_t)>& dr,
int type)
const {
39 auto d1 = sh1->discretizeBoundaryWithDensity(dr, type);
41 auto d2 = sh2->discretizeBoundaryWithDensity(dr, type);
43 return d1.subtract(
d2);
46 template <
typename vec_t>
48 const std::function<
scalar_t(vec_t)>& dr,
int internal_type,
int boundary_type)
const {
49 auto d1 = sh1->discretizeWithDensity(dr, internal_type, boundary_type);
51 auto d2 = sh2->discretizeBoundaryWithDensity(dr, boundary_type);
53 return d1.subtract(
d2);
56 template <
typename vec_t>
58 return os <<
"ShapeDifference(" << *sh1 <<
", " << *sh2 <<
")";
63 #endif // MEDUSA_BITS_DOMAINS_SHAPEDIFFERENCE_HPP_
static const shape_flags d1
Indicates to calculate d1 shapes.
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
static const shape_flags d2
Indicates to calculate d2 shapes.
DomainDiscretization< vec_t > discretizeWithStep(scalar_t step, int internal_type, int boundary_type) const override
Returns a discretization of this shape with approximately uniform distance step between nodes.
std::ostream & print(std::ostream &os) const override
Output information about this shape to given output stream os.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const override
Discretizes boundary with given density and fill engine.
DomainDiscretization< vec_t > discretizeWithDensity(const std::function< scalar_t(vec_t)> &dr, int internal_type, int boundary_type) const override
Returns a discretization of the domain with spatially variable step.
DomainDiscretization< vec_t > discretizeBoundaryWithStep(scalar_t step, int type) const override
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
vec_t::Scalar scalar_t
Scalar data type used in computation.