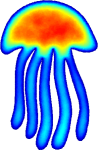 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
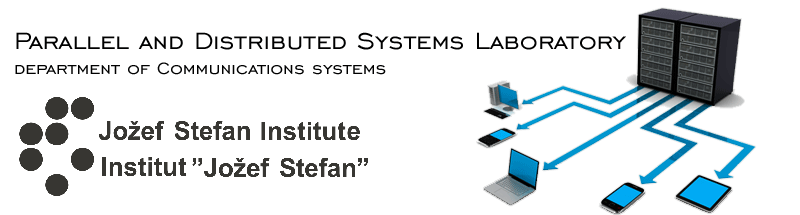 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_INTEGRATORS_RKEXPLICIT_FWD_HPP_
2 #define MEDUSA_BITS_INTEGRATORS_RKEXPLICIT_FWD_HPP_
19 template <
typename Scalar,
int num_steps>
31 template <
typename Scalar,
int num_stages>
38 Eigen::Matrix<scalar_t, stages, 1>
alpha_;
39 Eigen::Matrix<scalar_t, stages, stages>
beta_;
40 Eigen::Matrix<scalar_t, stages, 1>
gamma_;
56 RKExplicit(
const Eigen::Matrix<scalar_t, stages, 1>& alpha,
57 const Eigen::Matrix<scalar_t, stages, stages>& beta,
58 const Eigen::Matrix<scalar_t, stages, 1>& gamma)
64 template <
typename func_t>
88 std::forward_iterator_tag,
140 explicit operator bool() {
153 Eigen::VectorXd
value()
const {
return y; }
163 return os <<
"t = " <<
step.t <<
"; y = " <<
step.y;
185 return os <<
"Explicit RK integrator using " << integrator.
method_ <<
" method from "
186 <<
"time " << integrator.
t0_ <<
" with step " << integrator.
dt_ <<
" for "
203 template <
typename func_t>
205 const Eigen::VectorXd& y0)
const {
206 return Integrator<func_t>(*
this, func, t0, t_max, dt, y0);
211 template <
typename func_t>
212 Eigen::VectorXd
step(
const func_t& func,
scalar_t t,
const Eigen::VectorXd& y,
214 Eigen::Matrix<scalar_t, Eigen::Dynamic, stages> k(y.size(),
static_cast<int>(
stages));
215 k.col(0) = func(t +
alpha_(0) * dt, y);
216 for (
int i = 1; i <
stages; ++i) {
217 k.col(i) = func(t +
alpha_(i) * dt,
218 y + dt * k.leftCols(i) *
beta_.row(i).head(i).transpose());
220 return y + dt * k *
gamma_;
225 template <
typename scalar_t,
int stages>
229 template <
typename Scalar2,
int num_steps2>
234 template <
typename scalar_t,
int num_stages>
236 os <<
"RKExplicit with " << num_stages <<
" stages and "
237 <<
"alpha = " << method.
alpha_ <<
", "
238 <<
"beta = " << method.
beta_ <<
", "
239 <<
"gamma = " << method.
gamma_;
246 namespace integrators {
259 template <
class scalar_t =
double>
260 RKExplicit<scalar_t, 4>
RK4();
263 template <
class scalar_t =
double>
264 RKExplicit<scalar_t, 4>
RK38();
267 template <
class scalar_t =
double>
268 RKExplicit<scalar_t, 1>
Euler();
271 template <
class scalar_t =
double>
275 template <
class scalar_t =
double>
276 RKExplicit<scalar_t, 3>
RK3();
279 template <
class scalar_t =
double>
283 namespace of_order_internal {
284 template <
int order,
class scalar_t>
285 struct _of_order_impl {
static RKExplicit<scalar_t, order>
of_order(); };
292 template <
int order,
class scalar_t =
double>
301 #endif // MEDUSA_BITS_INTEGRATORS_RKEXPLICIT_FWD_HPP_
Root namespace for the whole library.
RKExplicit< scalar_t, 2 > Midpoint()
Explicit midpoint rule, 2nd order.
bool operator==(const IterationStep &other) const
Compare two steppers if they are on the same step.
Class representing an explicit Runge-Kutta method.
Integrator< func_t > solve(const func_t &func, scalar_t t0, scalar_t t_max, scalar_t dt, const Eigen::VectorXd &y0) const
Returns a solver using this method.
Integrator(const RKExplicit< scalar_t, num_stages > &method, const func_t &func, scalar_t t0, scalar_t t_max, scalar_t dt, Eigen::VectorXd y0)
Constructs stepper.
Class representing a step in the integration process.
Scalar scalar_t
Floating point type used in the computations.
const IterationStep & operator*() const
const version of IterationStep::operator*
bool operator!=(const IterationStep &other) const
Negation of IterationStep::operator==.
IterationStep operator++(int)
Advance the stepper for one time step, returning the old value.
RKExplicit< scalar_t, 3 > RK3()
Kutta's third order method.
bool is_last() const
Returns true if integrator just completed its last step.
static RKExplicit< scalar_t, order > of_order()
Returns Runge Kutta explicit method of requested order with given floating point type.
IterationStep & operator*()
Noop, used to conform to std::iterator requirements.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
IterationStep const_iterator
const iterator type
friend std::ostream & operator<<(std::ostream &os, const IterationStep &step)
Output current state of the stepper.
const func_t & func_
Function to integrate.
IterationStep iterator
iterator type
Eigen::VectorXd y0_
Initial value.
Eigen::Matrix< scalar_t, stages, 1 > gamma_
Bottom row of the tableau.
Eigen::VectorXd & value()
Read-write access to current value.
IterationStep(const Integrator &integrator)
Construct an iterator at the initial values of the equation.
friend std::ostream & operator<<(std::ostream &os, const RKExplicit< scalar_t, stages > &)
Output the method's tableau for debugging.
RKExplicit< scalar_t, 4 > RK38()
3/8 rule 4th order method
Integrator class for RK methods.
int iceil(T x)
Ceils a floating point to an integer.
friend std::ostream & operator<<(std::ostream &os, const Integrator &integrator)
Output information about this integrator.
const_iterator cend()
Same as Integrator::end()
const_iterator cbegin()
Same as Integrator::begin()
iterator end()
Creates an invalid stepper at positioned past the last step, meant to be used for comparison it == en...
IterationStep & operator++()
Advance the stepper for one time step, returning the new value.
IterationStep(const Integrator &integrator, int)
Construct an (invalid) iterator pointing past the last step.
iterator begin()
Creates stepper at positioned at initial value.
RKExplicit(const Eigen::Matrix< scalar_t, stages, 1 > &alpha, const Eigen::Matrix< scalar_t, stages, stages > &beta, const Eigen::Matrix< scalar_t, stages, 1 > &gamma)
Construct a Runge-Kutta method with tableau .
scalar_t & time()
Read-write access to current time.
int max_steps
Number of steps.
Eigen::VectorXd step(const func_t &func, scalar_t t, const Eigen::VectorXd &y, scalar_t dt) const
Returns next value.
RKExplicit< scalar_t, 4 > RK4()
Standard RK4 4th order method.
Eigen::Matrix< scalar_t, stages, 1 > alpha_
Left row of the tableau.
const Integrator & integrator
reference to underlying integrator
Eigen::VectorXd value() const
Get current value.
Eigen::VectorXd y
current value
Eigen::Matrix< scalar_t, stages, stages > beta_
Central matrix of the tableau.
Class representing an AdamsBashforth method, an explicit linear multistep method.
scalar_t time() const
Get current time.
RKExplicit< scalar_t, 6 > Fehlberg5()
Fifth order method appearing in Fehlberg's method.
const RKExplicit< scalar_t, num_stages > method_
Method used in this integrator.
RKExplicit< scalar_t, 1 > Euler()
Explicit Euler's method, 1st order.