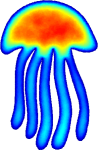 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
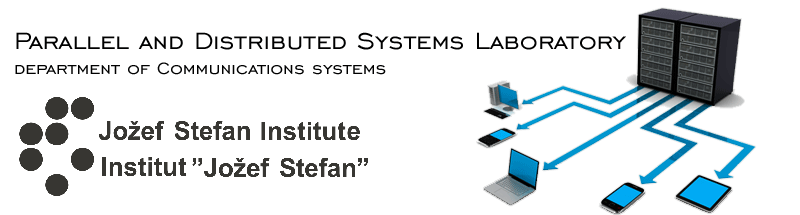 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_POLYHEDRONSHAPE_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_POLYHEDRONSHAPE_FWD_HPP_
16 #include <medusa/bits/domains/PolytopeShape.hpp>
19 #include <CGAL/AABB_face_graph_triangle_primitive.h>
20 #include <CGAL/AABB_traits.h>
21 #include <CGAL/AABB_tree.h>
22 #include <CGAL/Side_of_triangle_mesh.h>
23 #include <CGAL/Simple_cartesian.h>
24 #include <CGAL/Surface_mesh.h>
25 #include <CGAL/Polygon_mesh_processing/compute_normal.h>
45 template <
typename vec_t>
47 static_assert(
vec_t::dim == 3,
"Only available in 3 dimensions.");
51 typedef CGAL::Simple_cartesian<double>
K;
54 typedef CGAL::Surface_mesh<Point>
Mesh;
55 typedef Mesh::Vertex_index
VI;
56 typedef Mesh::Face_index
FI;
57 typedef CGAL::AABB_face_graph_triangle_primitive<Mesh>
Primitive;
60 typedef CGAL::AABB_tree<CGAL::AABB_traits<K, Primitive>>
Tree;
71 tree.accelerate_distance_queries();
73 auto vnormals =
surface.add_property_map<
VI,
Vector>(
"v:normals", CGAL::NULL_VECTOR).first;
74 auto fnormals =
surface.add_property_map<
FI,
Vector>(
"f:normals", CGAL::NULL_VECTOR).first;
75 CGAL::Polygon_mesh_processing::compute_normals(
surface, vnormals, fnormals);
111 std::ifstream input(filename);
112 assert_msg(input,
"Failed opening file %s: %s", filename, strerror(errno));
115 assert_msg(!input.fail(),
"Invalid OFF file - either parsing failed or the data "
116 "does not represent a two-manifold.");
117 assert_msg(input,
"Failed reading file %s: %s", filename, strerror(errno));
128 std::pair<vec_t, vec_t>
bbox()
const override {
129 auto b =
tree.bbox();
130 return {{b.xmin(), b.ymin(), b.zmin()}, {b.xmax(), b.ymax(), b.zmax()}};
134 const std::function<
scalar_t(vec_t)>& dr,
int type)
const override;
136 std::ostream&
print(std::ostream& os)
const override {
137 return os <<
"PolyhedronShape with " <<
surface.num_vertices() <<
" vertices"
138 <<
" and " <<
surface.num_faces() <<
" faces.";
144 static int rgb2type(uint8_t r, uint8_t g, uint8_t b) {
145 return -((
static_cast<int>(r) << 16) + (
static_cast<int>(g) << 8) + b) - 1;
152 return {p.x(), p.y(), p.z()};
165 template <
typename Scalar>
166 class PolytopeShape<
Vec<Scalar, 3>> :
public PolyhedronShape<Vec<Scalar, 3>> {
167 using base_t = PolyhedronShape<Vec<Scalar, 3>>;
168 using typename base_t::PolyhedronShape;
170 PolytopeShape(
const base_t& shape) : base_t(shape) {}
177 #endif // MEDUSA_BITS_DOMAINS_POLYHEDRONSHAPE_FWD_HPP_
Root namespace for the whole library.
PolyhedronShape & operator=(const PolyhedronShape &other)
Copy assignment (necessary to rebuild the tree).
static PolyhedronShape fromOFF(const std::string &filename)
Read a triangular closed surface mesh describing a polyhedron from a .off file.
Class representing domain discretization along with an associated shape.
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
PolyhedronShape * clone() const override
Polymorphic clone pattern.
@ dim
Number of elements of this matrix.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const override
Discretizes boundary with given density and fill engine.
@ dim
Dimensionality of the domain.
CGAL::AABB_face_graph_triangle_primitive< Mesh > Primitive
CGAL type for storing triangular faces of surface meshes in AABB tree.
#define assert_msg(cond,...)
Assert with better error reporting.
CGAL::AABB_tree< CGAL::AABB_traits< K, Primitive > > Tree
CGAL type for axis-aligned bounding-box trees, used for testing point inclusions.
CGAL::Side_of_triangle_mesh< Mesh, K > PointInside
CGAL type of the function for testing point inclusion.
Base class for geometric shapes of domains.
PolyhedronShape(const PolyhedronShape &other)
Copy constructor (necessary to rebuild the tree).
PolyhedronShape(const Mesh &surface)
Construct a shape from a CGAL surface mesh.
vec_t vector_t
Vector data type used in computations.
void init()
Initialize the tree and compute surface normals.
CGAL::Surface_mesh< Point > Mesh
CGAL surface mesh type.
virtual DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const =0
Discretizes boundary with given density and fill engine.
K::Point_3 Point
CGAL point type.
static int rgb2type(uint8_t r, uint8_t g, uint8_t b)
Convert a RBG color to an integer value that can be used as a boundary type.
PointInside inside_tester
Function used for testing point inclusions.
CGAL::Simple_cartesian< double > K
CGAL geometry kernel.
std::ostream & print(std::ostream &os) const override
Output information about this shape to given output stream os.
virtual DomainDiscretization< vec_t > discretizeBoundaryWithStep(scalar_t step, int type) const
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
Tree tree
AABB tree of the faces.
std::pair< vec_t, vec_t > bbox() const override
Return the bounding box of the domain.
K::Vector_3 Vector
CGAL vector type.
Mesh::Face_index FI
CGAL index type for a face on a surface mesh.
Mesh surface
Surface of the polyhedron represented as a triangular mesh.
Mesh::Vertex_index VI
CGAL index type for a point on a surface mesh.
A polyhedron represented by a closed triangular mesh.
vec_t::Scalar scalar_t
Scalar data type used in computation.
bool contains(const vec_t &point) const override
Return true if point is not more than margin() outside the domain.
vec_t getPoint(VI idx) const
Convert a CGAL vertex index to a Medusa vector.