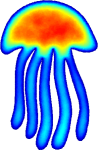 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
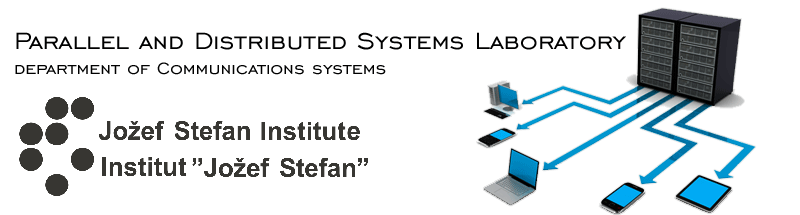 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_APPROXIMATIONS_POLYHARMONIC_HPP_
2 #define MEDUSA_BITS_APPROXIMATIONS_POLYHARMONIC_HPP_
14 template <
typename scal_t,
int k>
16 assert_msg(
order_ > 0,
"Order must be supplied if compile type order is -1.");
19 template <
typename scal_t,
int k>
24 template <
typename scal_t,
int k>
26 assert_msg(derivative >= 0,
"Derivative of negative order %d requested.", derivative);
31 while (--derivative >= 0) {
32 r *= (order_/2.0 - derivative);
37 template <
typename scal_t,
int k>
38 template <
int dimension>
40 assert_msg(order_ > 2,
"Order must be > 2 to compute the Laplacian, got %d.", k);
41 return (dimension + order_ - 2)*order_*
ipow(std::sqrt(r2), order_ - 2);
46 template <
class S,
int K>
48 return os <<
"Polyharmonic RBF of order " << b.
order_ <<
'.';
53 #endif // MEDUSA_BITS_APPROXIMATIONS_POLYHARMONIC_HPP_
const int order_
Exponent of the RBF.
Root namespace for the whole library.
T ipowneg(T b, int e)
Compute possibly negative integer power b^e.
Polyharmonic()
Default constructor. Only applicable when order given as template argument.
double ipow(double base)
Compile time integer power, returns base raised to power exponent.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
#define assert_msg(cond,...)
Assert with better error reporting.
int order() const
Get order of the RBF.
Polyharmonic Radial Basis Function of odd order.
Represents the Laplacian operator.
scalar_t operator()(scalar_t r2, int derivative) const
Evaluate derivative of this RBF wrt.
scal_t scalar_t
Scalar type used for computations.