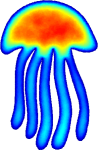 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
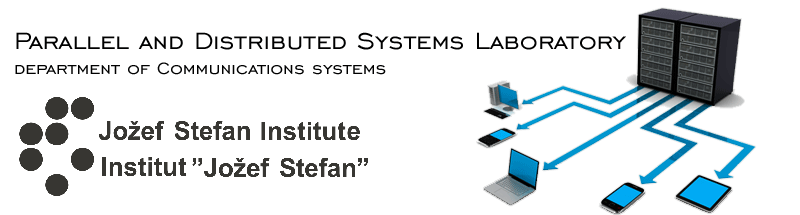 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_NURBSSHAPE_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_NURBSSHAPE_FWD_HPP_
23 namespace nurbs_shape_internal {
34 template <
typename vec_t,
typename param_vec_t>
51 template <
typename vec_t,
typename param_vec_t>
78 bool contains(
const vec_t& )
const override {
return true; }
82 std::pair<vec_t, vec_t>
bbox()
const override {
83 assert_msg(
false,
"This function is not available for this shape. A"
84 "bounding box of its DomainDiscretization should be taken.");
89 const std::function<
scalar_t(vec_t)>&,
int)
const override;
91 std::ostream&
print(std::ostream& ostream)
const override {
92 return ostream <<
"NURBS shape made of " <<
patches.size() <<
" patches.";
132 #endif // MEDUSA_BITS_DOMAINS_NURBSSHAPE_FWD_HPP_
Root namespace for the whole library.
NURBSShape & numSamples(int n_samples)
Controls the number of generated candidates from each point in surface fill.
Range< NURBSPatch< vec_t, param_vec_t > > patches
Range of NURBS patches.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
NURBSShape & proximityTolerance(scalar_t zeta)
Set proximity tolerance in surface fill.
Internal structure of NURBSShape that helps with partial class specialization.
@ dim
Number of elements of this matrix.
@ param_dim
Dimensionality of parametric space.
std::ostream & print(std::ostream &ostream) const override
Output information about this shape to given output stream os.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &, int) const override
Discretizes boundary with given density and fill engine.
#define assert_msg(cond,...)
Assert with better error reporting.
std::pair< vec_t, vec_t > bbox() const override
Return the bounding box of the domain.
Base class for geometric shapes of domains.
NURBSShape * clone() const override
Polymorphic clone pattern.
Class representing a single NURBS patch in an arbitrary dimensional space, defined on an arbitrary di...
NURBSShape & seed(int seed)
Set custom seed for the random number generator.
vec_t::scalar_t scalar_t
Scalar type.
bool hasContains() const override
Return true if shape has contains() method implemented.
Class representing a shape made out of NURBS patches in an arbitrary dimensional space.
NURBSShape(const Range< NURBSPatch< vec_t, param_vec_t >> &patches_in)
Construct NURBSShape from a Range of patches.
NURBSShape & maxPoints(int max_points)
Maximal number of points generated in surface fill.
int seed_
Seed for the random number generator.
scalar_t epsilon
Evaluate normals slightly away from the boundary.
int max_points
Maximal number of points generated in surface fill.
NURBSShape & boundaryProximity(scalar_t epsilon)
Calculate boundary normals epsilon times the size of domain away from the boundary.
@ dim
Dimensionality of space after projection.
scalar_t zeta
Proximity tolerance in surface fill.
@ proj_dim
Dimensionality of space before projection.
Vec< scalar_t, proj_dim > proj_vec_t
Vector type before projection.
An extension of std::vector<T> to support additional useful operations.
int n_samples
Number of samples in surface fill.
vec_t::Scalar scalar_t
Scalar data type used in computation.
bool contains(const vec_t &) const override
Return true if point is not more than margin() outside the domain.