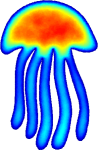 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
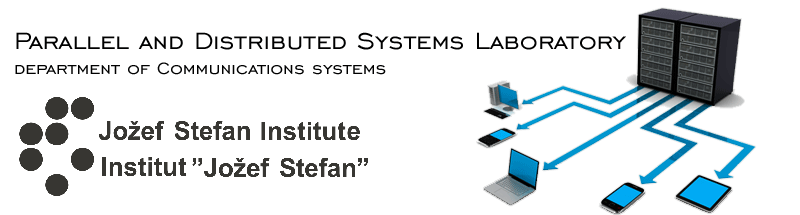 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_APPROXIMATIONS_MULTIQUADRIC_HPP_
2 #define MEDUSA_BITS_APPROXIMATIONS_MULTIQUADRIC_HPP_
16 template <
typename scal_t>
21 template <
class scal_t>
23 assert_msg(derivative >= 0,
"Derivative of negative order %d requested.", derivative);
26 for (
int i = 1; i < derivative; ++i) {
29 return c /
ipow(shape_, 2*derivative) / std::sqrt(
ipow(f+1, 2*derivative - 1));
32 template <
class scal_t>
33 template <
int dimension>
36 scal_t inverse = 1.0 / std::sqrt(1+f*r2);
37 return dimension*f*inverse - r2*f*f*
ipow(inverse, 3);
41 template <
class scal_t>
43 return std::sqrt(r2/shape_/shape_ + 1);
49 return os <<
"Multiquadric RBFs with shape " << b.
shape();
54 #endif // MEDUSA_BITS_APPROXIMATIONS_MULTIQUADRIC_HPP_
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
scalar_t shape() const
Returns shape parameter.
double ipow(double base)
Compile time integer power, returns base raised to power exponent.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
#define assert_msg(cond,...)
Assert with better error reporting.
scal_t scalar_t
Scalar type used for computations.
scalar_t operator()(scalar_t r2, int derivative) const
Evaluate derivative of this RBF wrt.
Multiquadric Radial Basis Function.
Multiquadric(scalar_t shape=1.0)
Creates a MultiQuadratic with shape parameter shape.
scalar_t shape_
Shape parameter.
Represents the Laplacian operator.