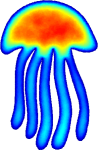 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
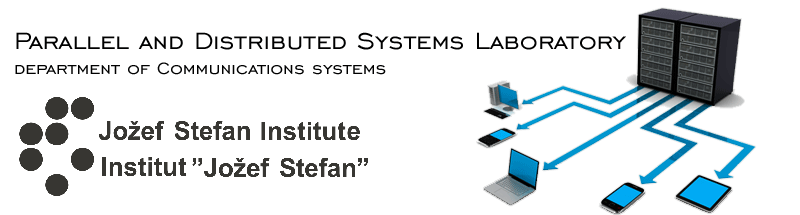 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_SPATIAL_SEARCH_KDTREEMUTABLE_FWD_HPP_
2 #define MEDUSA_BITS_SPATIAL_SEARCH_KDTREEMUTABLE_FWD_HPP_
13 #include <nanoflann/nanoflann.hpp>
35 template <
class vec_t>
42 static_assert(std::is_same<double, scalar_t>::value,
43 "Not Implemented Error: only implemented for double type!");
45 typedef nanoflann::KDTreeSingleIndexDynamicAdaptor<
46 nanoflann::L2_Simple_Adaptor<scalar_t, kdtree_internal::PointCloud<vec_t>>,
60 tree(
dim,
points_, nanoflann::KDTreeSingleIndexAdaptorParams(20)) {}
64 tree(
dim,
points_, nanoflann::KDTreeSingleIndexAdaptorParams(20)) {}
82 void insert(
const vec_t& point);
89 if (
size_ == 0)
return false;
90 return query(p).second[0] <= r*r;
123 #endif // MEDUSA_BITS_SPATIAL_SEARCH_KDTREEMUTABLE_FWD_HPP_
void insert(const vec_t &point)
Insert a point into the tree.
Root namespace for the whole library.
nanoflann::KDTreeSingleIndexDynamicAdaptor< nanoflann::L2_Simple_Adaptor< scalar_t, kdtree_internal::PointCloud< vec_t > >, kdtree_internal::PointCloud< vec_t >, dim > kd_tree_t
Tree type.
Scalar scalar_t
Type of the elements, alias of Scalar.
A k-d tree data structure that supports dynamic insertions and lazy-removal.
Helper class for KDTree with appropriate accessors containing a set of points.
std::pair< mm::Range< int >, mm::Range< double > > query(const vec_t &point, int k=1)
Find k nearest neighbors to given point.
friend std::ostream & operator<<(std::ostream &os, const KDTreeMutable< V > &tree)
Output basic info about given tree.
@ dim
Number of elements of this matrix.
void remove(int index)
Removes a point with given index from the tree.
vector_t::scalar_t scalar_t
Scalar type used.
vec_t vector_t
Vector type used.
@ dim
Dimensionality of the space.
bool existsPointInSphere(const vec_t &p, scalar_t r)
Check if any point exists in sphere centered at p with radius r.
void reset(const Range< vec_t > &points)
Grows a new tree with new points.
int size_
Number of points in the tree.
int size() const
Returns number of elements.
kd_tree_t tree
Actual tree build over points.
int size() const
Returns number of points in the tree.
kdtree_internal::PointCloud< vec_t > points_
Points, contained in the tree.
KDTreeMutable()
Creates an empty k-d tree.
KDTreeMutable(const Range< vec_t > &points)
Constructor that builds the search tree for the given points.