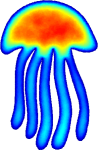 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
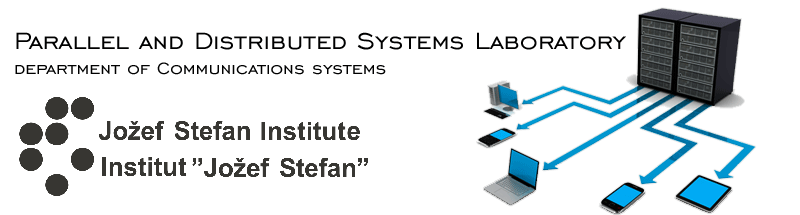 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_SPATIAL_SEARCH_POINTCLOUD_HPP_
2 #define MEDUSA_BITS_SPATIAL_SEARCH_POINTCLOUD_HPP_
14 namespace kdtree_internal {
17 template <
typename vec_t>
19 std::vector<vec_t>
pts;
41 inline vec_t
get(
const size_t idx)
const {
return pts[idx]; }
44 inline void add(
const vec_t& p) {
pts.push_back(p); }
54 #endif // MEDUSA_BITS_SPATIAL_SEARCH_POINTCLOUD_HPP_
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
vec_t get(const size_t idx) const
Access the points.
Helper class for KDTree with appropriate accessors containing a set of points.
PointCloud(const std::vector< vec_t > &pts)
Construct from an array of points.
@ dim
Number of elements of this matrix.
void setPts(const std::vector< vec_t > &pts)
Reset contained points.
std::vector< vec_t > pts
Points, contained in the tree.
void add(const vec_t &p)
Add a point to the cloud.
vec_t::scalar_t kdtree_get_pt(const size_t idx, int dim) const
Interface requirement: returns dim-th coordinate of idx-th point.
bool kdtree_get_bbox(BBOX &) const
Comply with the interface.
PointCloud()=default
Construct an empty point set.
int kdtree_get_point_count() const
Interface requirement: returns number of data points.