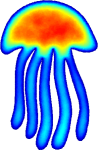 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
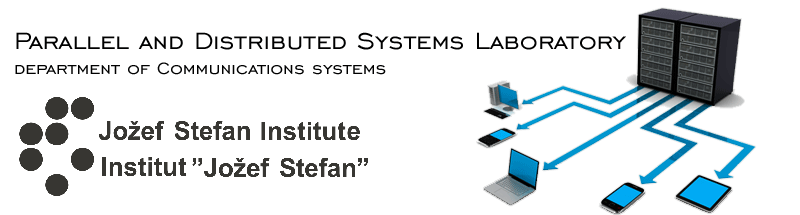 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_IMPLICITOPERATORS_HPP_
2 #define MEDUSA_BITS_OPERATORS_IMPLICITOPERATORS_HPP_
14 template <
class shape_storage_type,
class matrix_type,
class rhs_type>
17 ss(&ss), M(&M), rhs(&rhs), row_offset(row_offset), col_offset(col_offset) {
21 "Matrix does not have enough rows, expected at least %d = %d + %d, got %d.",
24 "Matrix does not have enough columns, expected at least %d = %d + %d, got %d.",
27 "RHS vector does not have enough rows, expected at least %d = %d + %d, got %d.",
31 if (
M.squaredNorm() > 1e-10) {
32 std::cerr <<
"Warning: matrix in implicit operators not initialized to zero!" << std::endl;
34 if (
rhs.squaredNorm() > 1e-10) {
35 std::cerr <<
"Warning: rhs in implicit operators not initialized to zero!" << std::endl;
40 template <
class S,
class matrix_type,
class rhs_type>
43 "Matrix does not have enough rows, expected at least %d, got %d.",
44 ss->size(), M->rows());
46 "Matrix does not have enough columns, expected at least %d, got %d.",
47 ss->size(), M->cols());
48 assert_msg(v == v,
"You tried to set M(%d, %d) to NaN. Check your computed shapes.",
49 row_offset+i, col_offset+j);
50 M->coeffRef(i+row_offset, j+col_offset) += v;
53 template <
class shape_storage_type,
class matrix_type,
class rhs_type>
56 "RHS vector does not have enough rows, expected at least %d, got %d.",
57 ss->size(), rhs->size());
58 assert_msg(v == v,
"You tried to set rhs(%d) to NaN. Check your computed shapes.",
60 rhs->operator[](i+row_offset) += v;
64 template <
typename S,
typename M,
typename R>
66 os <<
"Implicit operators ";
67 os << ((op.
hasShapes()) ?
"with" :
"without") <<
" linked storage ";
69 os <<
"without linked storage";
71 os <<
"over storage: " << *op.
ss;
73 os <<
" with " << ((op.
hasMatrix()) ?
"defined" :
"undefined") <<
" problem matrix";
74 os <<
" and with " << ((op.
hasRhs()) ?
"defined" :
"undefined") <<
" problem rhs.";
79 template <
typename Derived,
typename vec_t,
typename OpFamilies>
80 template <
typename M,
typename R>
81 ImplicitOperators<Derived, M, R>
83 return {*
static_cast<const Derived*
>(
this), matrix, rhs};
89 #endif // MEDUSA_BITS_OPERATORS_IMPLICITOPERATORS_HPP_
Root namespace for the whole library.
rhs_type rhs_t
Right hand side type.
const shape_storage_t * ss
Shape storage, but name is shortened for readability.
void addToRhs(int i, scalar_t v)
Adds v to rhs[i].
matrix_t::Scalar scalar_t
Scalar type of matrix elements.
ImplicitOperators()
Default constructor sets offset to 0 and pointers to nullptr.
rhs_t * rhs
Pointer to right hand side.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
#define assert_msg(cond,...)
Assert with better error reporting.
ImplicitOperators< Derived, M, R > implicitOperators(M &matrix, R &rhs) const
Construct implicit operators over this storage.
bool hasMatrix() const
Returns true if operators have a non-null pointer to problem matrix.
matrix_t * M
Pointer to problem matrix.
bool hasShapes() const
Returns true if operators have a non-null pointer to storage.
matrix_type matrix_t
Matrix type.
This class represents implicit operators that fill given matrix M and right hand side rhs with approp...
int row_offset
Row offset to be used when accessing matrix or rhs coefficients.
bool hasRhs() const
Returns true if operators have a non-null pointer to problem right hand side.
void addToM(int i, int j, scalar_t v)
Add v to M(i, j).
shape_storage_type shape_storage_t
Type of shape storage.
int col_offset
Column offset to be used when accessing matrix coefficients.