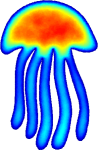 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
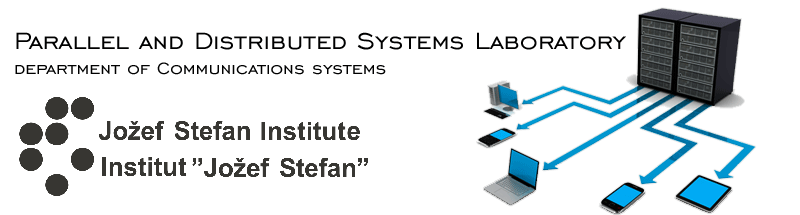 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_SPATIAL_SEARCH_GRID_HPP_
2 #define MEDUSA_BITS_SPATIAL_SEARCH_GRID_HPP_
16 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
18 assert_msg(0 <= i && i <
dim,
"Dimension index %i must be in range [0, %d)", i,
dim);
22 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
25 assert_msg(inBounds(index, sizes_),
"Index %s is out of bounds %s.", index, sizes_);
26 return data_[linearIndex(index, sizes_)];
29 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
31 assert_msg(inBounds(index, sizes_),
"Index %s is out of bounds %s.", index, sizes_);
32 return data_[linearIndex(index, sizes_)];
35 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
37 assert_msg(0 <= index && index < size_,
"Index %d is out of bounds [0, %d).", index, size_);
41 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
43 assert_msg(0 <= index && index < size_,
"Index %d is out of bounds [0, %d).", index, size_);
47 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
50 for (
int i = 0; i <
dim; ++i) {
56 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
59 for (
int i = 0; i <
dim; ++i) {
60 if (index[i] < 0 || index[i] >= bounds[i])
return false;
65 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
68 assert_msg(inBounds(index, bounds),
"Index %s is out of bounds %s.", index, bounds);
70 for (
int i = 0; i <
dim; ++i) {
77 template <
typename T,
int dimension,
typename IndexType,
typename IndexArrayT>
81 for (
int i =
dim-1; i >= 0; --i) {
82 multi_index[i] = index % bounds[i];
89 template <
typename T,
int dim,
typename I,
typename IA>
91 os <<
dim <<
"D grid with sizes " << grid.
sizes_ <<
" and " << grid.
size_ <<
" elements, ";
99 #endif // MEDUSA_BITS_SPATIAL_SEARCH_GRID_HPP_
Root namespace for the whole library.
const T & operator()(const IndexArray &index) const
Readonly access to the grid.
IndexArray multiIndex(const Index &index) const
Compute multi-index from given linear index.
int Index
Type of the index used.
@ dim
Number of elements of this matrix.
Index size() const
Get total number of elements.
static Index computeSize(const IndexArray &sizes)
Compute the number of elements.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
const T & operator[](const Index &index) const
Readonly access to the grid.
#define assert_msg(cond,...)
Assert with better error reporting.
Class representing a simple n-dimensional grid structure, which supports indexing and storing values.
Index linearIndex(const IndexArray &index) const
Compute linear index from given multi-index.
IndexArray sizes_
Boundary of the grid in all dimensions.
const std::vector< T > & data() const
Get read-only access to linear data.
IndexArrayT IndexArray
Multiindex type.
bool inBounds(const IndexArray &index) const
Check if given index in in bounds.
IndexType size_
Total number of grid cells.
std::size_t mem_used(const container_t &v)
Returns number of bytes the container uses in memory.
std::string mem2str(std::size_t bytes)
Simple function to help format memory amounts for printing.