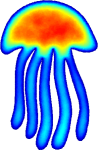 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
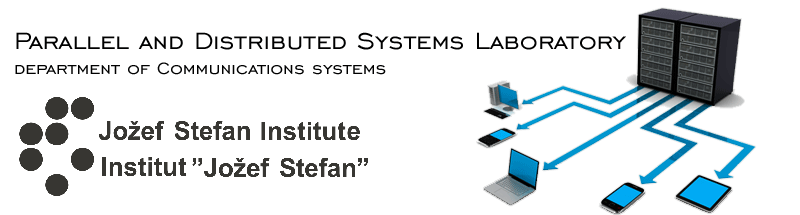 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_SPATIAL_SEARCH_GRID_FWD_HPP_
2 #define MEDUSA_BITS_SPATIAL_SEARCH_GRID_FWD_HPP_
34 template <
typename T,
int dimension,
typename IndexType = int,
35 typename IndexArrayT = std::array<IndexType, dimension>>
40 enum {
dim = dimension };
63 const std::vector<T>&
data()
const {
return data_; }
101 template <
typename U,
int D,
typename I,
typename IA>
107 #endif // MEDUSA_BITS_SPATIAL_SEARCH_GRID_FWD_HPP_
Grid(const IndexArray &sizes, const T &value)
Construct a grid with given sizes initialized to value.
Root namespace for the whole library.
const T & operator()(const IndexArray &index) const
Readonly access to the grid.
IndexArray multiIndex(const Index &index) const
Compute multi-index from given linear index.
IndexType Index
Type of the index used.
Index size() const
Get total number of elements.
static Index computeSize(const IndexArray &sizes)
Compute the number of elements.
const T & operator[](const Index &index) const
Readonly access to the grid.
friend std::ostream & operator<<(std::ostream &os, const Grid< U, D, I, IA > &grid)
Output some information about given grid.
std::vector< T > data_
Data stored in the grid.
Class representing a simple n-dimensional grid structure, which supports indexing and storing values.
std::vector< T > & data()
Get read-write access to linear data.
Index linearIndex(const IndexArray &index) const
Compute linear index from given multi-index.
IndexArray sizes_
Boundary of the grid in all dimensions.
const std::vector< T > & data() const
Get read-only access to linear data.
IndexArrayT IndexArray
Multiindex type.
bool inBounds(const IndexArray &index) const
Check if given index in in bounds.
IndexType size_
Total number of grid cells.
T value_type
Type of the values stored in the grid.
@ dim
Dimensionality of the domain.
IndexArray sizes() const
Get grid sizes.
Grid(const IndexArray &sizes)
Construct a zero initialized grid with given sizes.