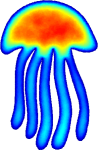 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
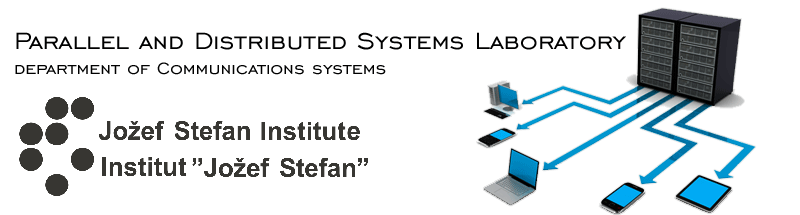 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_FINDCLOSEST_HPP_
2 #define MEDUSA_BITS_DOMAINS_FINDCLOSEST_HPP_
20 template <
typename domain_t>
24 if (for_which.empty()) for_which = domain.all();
26 if (search_among.empty()) {
30 assert_msg(!domain.positions().empty(),
"Cannot find support in an empty domain.");
33 "Support size (%d) cannot exceed number of points that we are searching among (%d).",
35 assert_msg(!for_which.empty(),
"Set of nodes for which to find the support is empty. "
36 "There appear to be no nodes in domain discretization.");
37 assert_msg(!search_among.empty(),
"Set of nodes to search among is empty. "
38 "There appear to be no nodes in domain discretization.");
39 for (
int x : for_which) {
40 assert_msg(0 <= x && x < domain.size(),
"Index %d out of range [%d, %d) in forNodes.",
43 for (
int x : search_among) {
44 assert_msg(0 <= x && x < domain.size(),
"Index %d out of range [%d, %d) in "
45 "searchAmong.", x, 0, domain.size());
49 for (
int i : for_which) {
52 domain.support(i) = {i};
53 domain.support(i).insert(domain.support(i).end(), ss.begin(), ss.end()-1);
55 domain.support(i) = ss;
59 for (
int i : for_which) {
67 #endif // MEDUSA_BITS_DOMAINS_FINDCLOSEST_HPP_
Root namespace for the whole library.
Class representing a static k-d tree data structure.
void operator()(domain_t &domain) const
Find support for nodes in domain.
static Range seq(V n)
Returns range {0, ..., n-1}.
#define assert_msg(cond,...)
Assert with better error reporting.
std::pair< Range< int >, Range< scalar_t > > query(const vec_t &point, int k=1) const
Find k nearest neighbors to given point.
int support_size
Support size.
Range< int > search_among_
Search only among these nodes.
Range< int > for_which_
Find support only for these nodes.
bool force_self_
Force each node as the first element of its support.