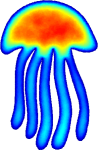 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
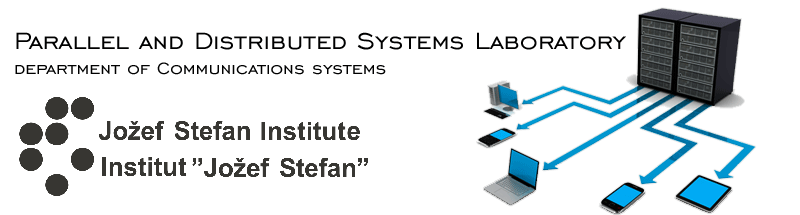 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_FINDCLOSEST_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_FINDCLOSEST_FWD_HPP_
51 template <
typename domain_t>
57 #endif // MEDUSA_BITS_DOMAINS_FINDCLOSEST_FWD_HPP_
Root namespace for the whole library.
FindClosest & numClosest(int num)
Find num closest nodes. This methods overrides the value set in the constructor.
void operator()(domain_t &domain) const
Find support for nodes in domain.
FindClosest & searchAmong(indexes_t search_among)
Search only among given nodes.
FindClosest & forNodes(indexes_t for_which)
Find support only for these nodes.
int support_size
Support size.
Class representing the engine for finding supports consisting of closest nodes.
std::vector< int > indexes_t
Class representing a collection of indices.
Range< int > search_among_
Search only among these nodes.
Range< int > for_which_
Find support only for these nodes.
FindClosest(int support_size)
Constructs an engine for finding support with given support size.
bool force_self_
Force each node as the first element of its support.
FindClosest & forceSelf(bool b=true)
Put each node as the first of its support, even if it is not included in searchAmong().