§ Features
§ Expressiveness and simplicity
Medusa aims to express the mathematical model in C++ code as intuitively, clearly and concisely as possible. Apart from the demo on the home page, three more examples are featured here.
§ Advection-diffusion equation
First, let's look at a steady state advection-diffusion equation: $$8 \, (-1, -1, -1) \cdot \nabla u + 2 \, \nabla^2 u = -1$$ on a domain with a hole with Dirichlet boundary conditions $u = 0$. Compare the above equation to the C++ code:
for (int i : domain.interior()) { 8.0*op.grad(i, {-1, -1, -1}) + 2.0*op.lap(i) = -1.0; } for (int i : domain.boundary()) { op.value(i) = 0.0; }
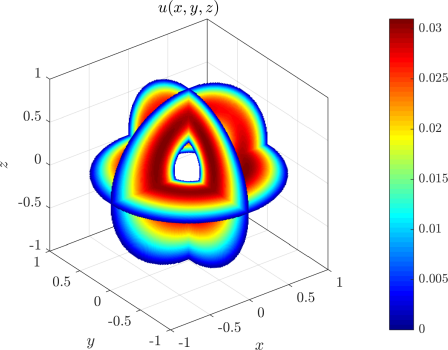
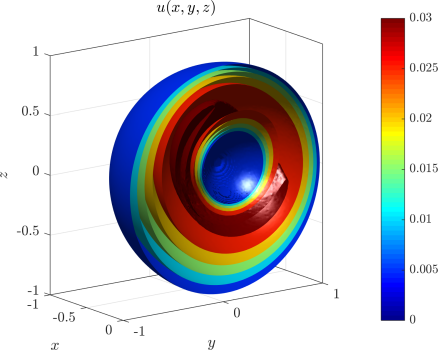
§ Navier-Stokes equations
§ Cantilever beam
§ Simple analyses of various setups
Many common strong-form mesh-free approximations can be seamlessly changed: discrete least squares, collocation, Radial Basis Function-generated finite differences, monomial augmentation, etc.
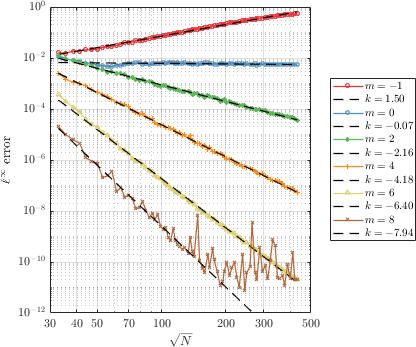
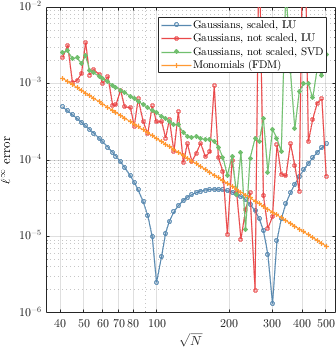
Approximations can be easily defined and changed. You can control types of RBFs, dimension of the space, monomial augmentation, shape parameters, scaling, system solvers...
RBFFD<Polyharmonic<double>, Vec2d, ScaleToClosest> approx(3, Monomials<Vec2d>(2)); WLS<MQs<Vec3d>, GaussianWeight<Vec3d>, NoScale, Eigen::PartialPivLU<Eigen::MatrixXd>> wls({9, 1.5}, 0.8);
§ Node generation
We support variable density node generation in any dimension with various node generation algorithms and spatial search structures as well as post processing iterative improvement techniques. However, you can also generate regularly spaced nodes on a unit square, for example.
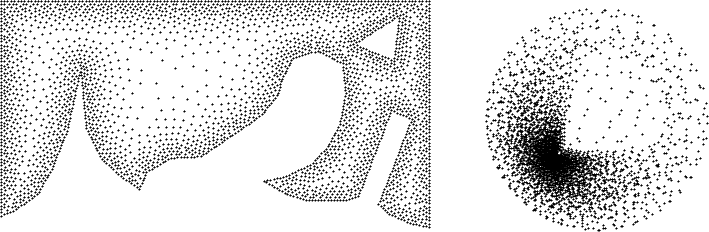
§ Dimension independence
Our aim is to have the code as dimension agnostic as possible. This is why most of our code, such as the node generation algorithms, approximation methods and solution procedures work in arbitrary dimensions.
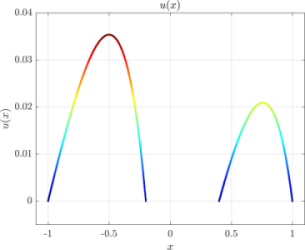
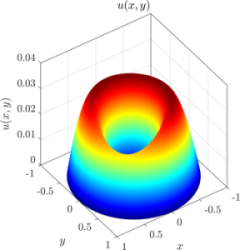
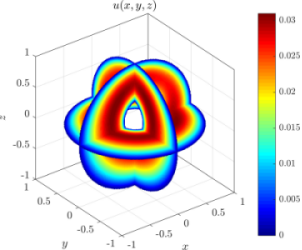
The solution of the advection-diffusion equation from the top of the page is shown here, using the same code as above in all dimensions. The source code is available here along with other Medusa examples. If care is taken, the dimension of the problem can be a runtime parameter.
§ Utilities
The library is divided into modules, whose precise contents are listed in the modules page of the API reference. Main parts are showcased here.
§ Domains
The library directly supports circular and rectangular domains in all three dimensions. Additionally, We support 2D polygons and arbitrary union/difference/affine transformation of all these. We also support parametrically given domains (including NURBS). Any such domain can be discretized with respect to any nodal spacing function or a discretization obtained elsewhere can be loaded from a file.
§ Approximations
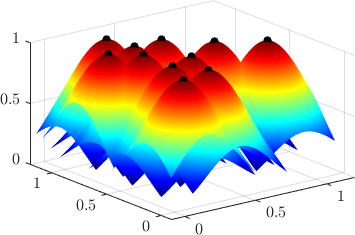
Many common strong-form mesh-free approximations can be seamlessly changed: discrete least squares, collocation, Radial Basis Functions generated finite differences, monomial augmentation … Every part of the approximation procedure is modular and fully customizable.
§ Operators
Discretized differential operators in Medusa allow for elegant code that very closely resembles the mathematical formulation. Nonetheless, the user has access to coordinate derivatives to assemble any operator and to all fields and linear systems involved.
§ Miscellaneous
Other convenience features such as fast spatial search structures (static and dynamic), time measuring utilities, and input and output to HDF, CSV and XML files.§ Extensibility
The library is designed with extensibility and multipurpose use in mind. It has been shaped through the use in different projects and each part of the library is designed to be usable on its own without any hard dependencies. You can define you own operators, add another radial basis function, new domain shapes or use just theTimer
class.