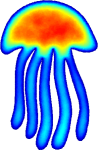 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
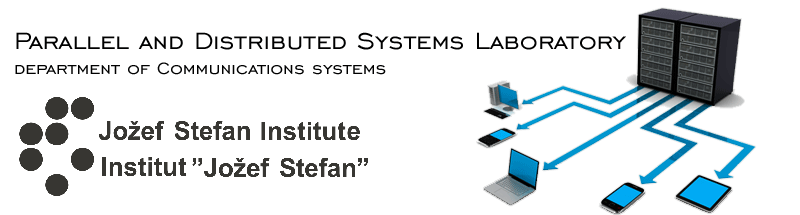 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_CAD_HELPERS_HPP_
2 #define MEDUSA_BITS_DOMAINS_CAD_HELPERS_HPP_
15 namespace cad_helpers {
17 template <
typename scalar_t,
int dim>
21 if (t == *(knots.end() - 1)) {
22 return *(control_points.end() - 1);
23 }
else if (t == *knots.begin()) {
24 return *control_points.begin();
28 "Not enough knots or parameter not within range. Try padding the knot vector p times.");
33 for (
int r = 1; r < p + 1; r++) {
34 for (
int j = p; j > r - 1; j--) {
35 scalar_t alpha = (t - knots[j + k - p]) / (knots[j + 1 + k - r] - knots[j + k - p]);
36 d[j] = (1.0 - alpha) * d[j - 1] + alpha * d[j];
43 template <
typename scalar_t,
int dim>
47 if (t > knots[knots.
size() - 1] && abs(t - knots[knots.
size() - 1]) < epsilon) {
48 t = knots[knots.
size() - 1];
49 }
else if (t < knots[0] && abs(t - knots[0]) < epsilon) {
54 int k = std::upper_bound(knots.begin(), knots.end(), t) - knots.begin();
60 template <
typename scalar_t,
int dim>
68 template <
typename scalar_t,
int dim>
73 der_control_points.resize(control_points.size() - 1);
76 for (
int i = 0; i < control_points.size() - 1; i++) {
78 for (
int j =0; j <
dim; j++) {
79 der_control_points[i](j) = temp * (control_points[i + 1](j) - control_points[i](j));
84 template <
typename scalar_t>
88 der_knots.resize(knots.
size() - 2);
91 for (
int i = 0; i < der_knots.
size(); i++) {
92 der_knots[i] = knots[i + 1];
99 #endif // MEDUSA_BITS_DOMAINS_CAD_HELPERS_HPP_
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
void generate_b_spline_derivative_control_points(int p, const Range< Vec< scalar_t, dim >> &control_points, const Range< scalar_t > &knots, Range< Vec< scalar_t, dim >> &der_control_points)
Generate control points of a B-spline that is the first derivative of the inputed B-spline.
@ dim
Number of elements of this matrix.
#define assert_msg(cond,...)
Assert with better error reporting.
void generate_b_spline_derivative(int p, const Range< Vec< scalar_t, dim >> &control_points, const Range< scalar_t > &knots, Range< Vec< scalar_t, dim >> &der_control_points, Range< scalar_t > &der_knots)
Generate control points and knot vector of a B-spline that is the first derivative of the inputed B-s...
void generate_b_spline_derivative_knots(const Range< scalar_t > &knots, Range< scalar_t > &der_knots)
Generate knots of a B-spline that is the first derivative of the inputed B-spline.
Vec< scalar_t, dim > evaluate_b_spline(scalar_t t, int p, const Range< Vec< scalar_t, dim >> &control_points, const Range< scalar_t > &knots, int k)
Evaluate B-spline in one point using De Boor's algorithm - , where is the number of dimensions.
int size() const
Returns number of elements.
An extension of std::vector<T> to support additional useful operations.