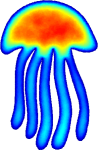 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
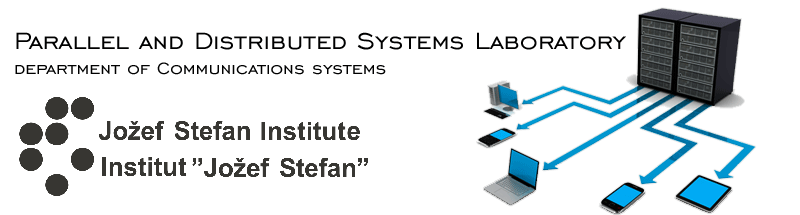 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_TYPES_VECTORFIELD_HPP_
2 #define MEDUSA_BITS_TYPES_VECTORFIELD_HPP_
13 template <
typename Scalar,
int dimension>
14 template <
typename OtherDerived>
19 template <
typename Scalar,
int dimension>
20 template <
typename OtherDerived>
22 const Eigen::MatrixBase<OtherDerived>& other) {
27 template <
typename Scalar,
int dimension>
28 VectorField<Scalar, dimension>&
30 Base::rowwise() = v.transpose();
35 template <
typename Scalar,
int dimension>
36 template <
typename OtherDerived>
38 const Eigen::PlainObjectBase<OtherDerived>& other) {
39 assert_msg(other.size() % dimension == 0,
"The length of the given data is not a "
40 "multiple of %d.", dimension);
41 return Eigen::Map<const Eigen::Matrix<Scalar, Eigen::Dynamic, dimension>, 0>(
42 other.data(), other.size()/dimension, dimension);
48 #endif // MEDUSA_BITS_TYPES_VECTORFIELD_HPP_
Matrix & operator=(const Scalar &s)
Assign scalar to a matrix, setting all its elements to s.
Root namespace for the whole library.
Eigen::Matrix< Scalar, Eigen::Dynamic, dimension > Base
Base class.
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
static VectorField fromLinear(const Eigen::PlainObjectBase< OtherDerived > &other)
Construct VectorField from its linear representation, obtained from asLinear().
VectorField(void)
Construct a vector field of size 0.
#define assert_msg(cond,...)
Assert with better error reporting.
Represents a discretization of a vector field, a finite collection of vectors.
VectorField & operator=(const VectorField &)=default
Default copy assignment.