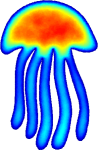 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
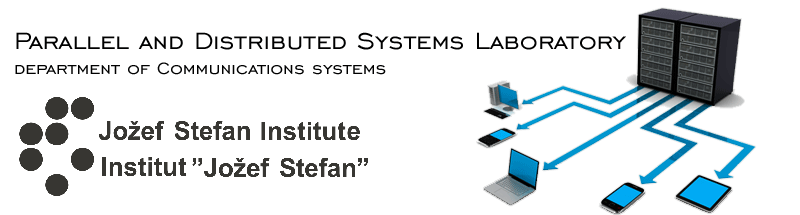 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_TYPES_VECTORFIELD_FWD_HPP_
2 #define MEDUSA_BITS_TYPES_VECTORFIELD_FWD_HPP_
37 template <
typename Scalar,
int dimension>
41 typedef Eigen::Matrix<Scalar, Eigen::Dynamic, dimension>
Base;
42 using Base::operator=;
56 template <typename OtherDerived>
57 VectorField(const Eigen::MatrixBase<OtherDerived>& other);
60 template <typename OtherDerived>
61 VectorField& operator=(const Eigen::MatrixBase<OtherDerived>& other);
67 template <typename SizeType>
74 template <
typename OtherDerived>
94 Eigen::Transpose<typename Base::RowXpr>
operator[](
typename Eigen::Index i) {
95 return Base::row(i).transpose();
98 SINL Eigen::Transpose<typename Base::ConstRowXpr>
operator[](
typename Eigen::Index i)
const {
99 return Base::row(i).transpose();
102 using Base::operator();
104 SINL Eigen::Transpose<typename Base::RowXpr>
operator()(
typename Eigen::Index i) {
105 return Base::row(i).transpose();
108 SINL Eigen::Transpose<typename Base::ConstRowXpr>
operator()(
typename Eigen::Index i)
const {
109 return Base::row(i).transpose();
116 SINL typename Base::ColXpr
c(
typename Eigen::Index i) {
return Base::col(i); }
118 SINL typename Base::ConstColXpr
c(
typename Eigen::Index i)
const {
return Base::col(i); }
121 Eigen::IndexedView<Base, indexes_t, Eigen::internal::AllRange<dimension>>
123 return Base::operator()(rowIndices,
Eigen::all);
132 Scalar*
begin() {
return this->data(); }
134 const Scalar*
begin()
const {
return this->data(); }
137 Scalar*
end() {
return this->data() + this->size(); }
139 const Scalar*
end()
const {
return this->data() + this->size(); }
142 const Scalar*
cbegin()
const {
return this->data(); }
144 const Scalar*
cend()
const {
return this->data() + this->size(); }
157 #endif // MEDUSA_BITS_TYPES_VECTORFIELD_FWD_HPP_
const indexes_t & indexes
Indexes of this view.
Root namespace for the whole library.
Eigen::Matrix< Scalar, Eigen::Dynamic, dimension > Base
Base class.
SINL Eigen::Transpose< typename Base::RowXpr > operator()(typename Eigen::Index i)
Return a single row as a vector, non-const version.
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
Eigen::IndexedView< Base, indexes_t, Eigen::internal::AllRange< dimension > > SINL operator()(const indexes_t &rowIndices)
Return an indexed view to a subset of vectors.
const Scalar * end() const
Const pointer to the last coefficient.
SINL Base::ColXpr c(typename Eigen::Index i)
Return the i-th component of this vector field.
static VectorField fromLinear(const Eigen::PlainObjectBase< OtherDerived > &other)
Construct VectorField from its linear representation, obtained from asLinear().
const Scalar * begin() const
Pointer to the first coefficient.
Vec< Scalar, dim > type
Underlying vector type.
@ dim
Number of elements of this matrix.
VectorField(void)
Construct a vector field of size 0.
VectorField< double, 3 > VectorField3d
Three dimensional vector field of doubles.
Scalar * end()
Pointer to the last coefficient.
Eigen::Transpose< typename Base::RowXpr > operator[](typename Eigen::Index i)
Return a single row as a vector, non-const version.
SINL Base::ConstColXpr c(typename Eigen::Index i) const
Const version of VectorField::c().
VectorField< double, 1 > VectorField1d
One dimensional vector field of doubles.
static const shape_flags all
Indicates to prepare all shapes, default.
const Scalar * cend() const
Const version of end.
std::vector< int > indexes_t
Class representing a collection of indices.
SINL Eigen::Transpose< typename Base::ConstRowXpr > operator[](typename Eigen::Index i) const
Return a single row as a vector, const version.
Scalar scalar_t
Scalar data type.
VectorFieldView(VectorField &sf, const indexes_t &indexes)
Construct a non contiguous view to a scalar field.
const Scalar * cbegin() const
Const version of begin.
Type trait for vector fields to obtain their underlying vector type.
Represents a discretization of a vector field, a finite collection of vectors.
VectorField< double, 2 > VectorField2d
Two dimensional vector field of doubles.
SINL Eigen::Transpose< typename Base::ConstRowXpr > operator()(typename Eigen::Index i) const
Return a single row as a vector, const version.
VectorField & sf
Reference to the viewed field.
Scalar * begin()
Pointer to the first coefficient.
#define SINL
Strong inline macro.
VectorField & operator=(const VectorField &)=default
Default copy assignment.
SINL VectorFieldView operator[](const indexes_t &indexes)
Returns an indexed view to a subset of vectors, that can be assigned.
Matrix(const Scalar &s)
Construct matrix from scalar. Enabled only for fixed size matrices.
void operator=(const Vec< scalar_t, dimension > &other)
Assign a vector to this view.
Represents a non contiguous view to a vector field.