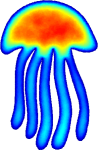 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
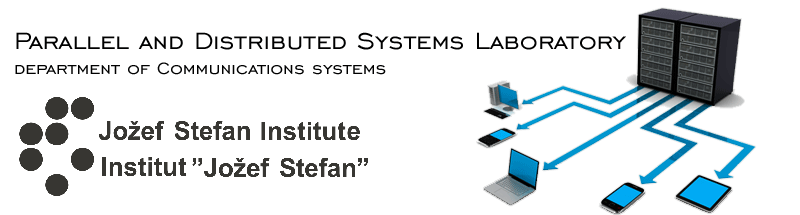 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_TRANSLATEDSHAPE_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_TRANSLATEDSHAPE_FWD_HPP_
26 template <
typename vec_t>
45 bool contains(
const vec_t& point)
const override {
46 return sh->contains(point-
a);
51 std::pair<vec_t, vec_t>
bbox()
const override {
53 return {bb.first+
a, bb.second+
a};
58 std::ostream&
print(std::ostream& os)
const override {
59 return os <<
"Shape " << *
sh <<
" translated by " <<
a;
65 int boundary_type)
const override;
68 const std::function<
scalar_t(vec_t)>& dr,
int internal_type,
69 int boundary_type)
const override;
72 const std::function<
scalar_t(vec_t)>& dr,
int type)
const override;
83 #endif // MEDUSA_BITS_DOMAINS_TRANSLATEDSHAPE_FWD_HPP_
std::pair< vec_t, vec_t > bbox() const override
Return the bounding box of the domain.
Root namespace for the whole library.
bool contains(const vec_t &point) const override
Return true if point is not more than margin() outside the domain.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
DomainDiscretization< vec_t > discretizeWithDensity(const std::function< scalar_t(vec_t)> &dr, int internal_type, int boundary_type) const override
Returns a discretization of the domain with spatially variable step.
deep_copy_unique_ptr< DomainShape< vec_t > > sh
Shape to be translated.
Class for working with translated domain shapes.
vec_t a
Translation vector.
const DomainShape< vec_t > & shape() const
Returns the underlying shape.
Base class for geometric shapes of domains.
vec_t translation() const
Returns the translation vector.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const override
Discretizes boundary with given density and fill engine.
bool hasContains() const override
Return true if shape has contains() method implemented.
TranslatedShape(const DomainShape< vec_t > &sh, const vec_t &a)
Construct a translated shape by specifying a shape and a translation vector.
std::ostream & print(std::ostream &os) const override
Output information about this shape to given output stream os.
DomainDiscretization< vec_t > discretizeBoundaryWithStep(scalar_t step, int type) const override
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
DomainDiscretization< vec_t > discretizeWithStep(scalar_t step, int internal_type, int boundary_type) const override
Returns a discretization of this shape with approximately uniform distance step between nodes.
Unique pointer with polymorphic deep copy semantics.
vec_t::Scalar scalar_t
Scalar data type used in computation.
TranslatedShape< vec_t > * clone() const override
Polymorphic clone pattern.