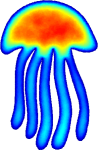 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
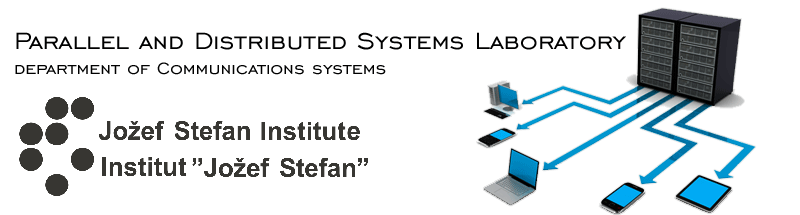 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_INTERPOLANTS_SHEPPARD_FWD_HPP_
2 #define MEDUSA_BITS_INTERPOLANTS_SHEPPARD_FWD_HPP_
35 template <
class vec_t,
class value_t>
55 assert_msg(N > 0,
"Number of positions in the tree must be greater than 0, got %d.", N);
68 assert_msg(N > 0,
"Number of positions in the tree must be greater than 0, got %d.", N);
70 "Number of positions must equal number of values. Got %d positions in the tree "
79 template <
typename values_container_t>
80 void setValues(
const values_container_t& new_values) {
104 value_t
operator()(
const vec_t& point,
int num_closest,
int power = 2,
double reg = 0.0,
105 double conf_dist = 1e-12)
const;
110 #endif // MEDUSA_BITS_INTERPOLANTS_SHEPPARD_FWD_HPP_
Range< value_t > values
Function values at given points.
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing a static k-d tree data structure.
value_t operator()(const vec_t &point, int num_closest, int power=2, double reg=0.0, double conf_dist=1e-12) const
Evaluate the interpolant at the given point.
#define assert_msg(cond,...)
Assert with better error reporting.
KDTree< vec_t > tree
Tree of all points.
SheppardInterpolant(const Range< vec_t > &pos, const Range< value_t > &values)
Construct a new Sheppard Interpolant object.
Scattered interpolant using a slightly modified Sheppard's interpolation (inverse distance weighting)...
vec_t::scalar_t scalar_t
Scalar data type.
int size() const
Returns number of elements.
SheppardInterpolant()=default
Construct a new empty Sheppard Interpolant object.
void setPositions(const Range< vec_t > &pos)
Set new positions.
void setValues(const values_container_t &new_values)
Set new values.
SheppardInterpolant(const Range< vec_t > &pos)
Construct a new Sheppard Interpolant.