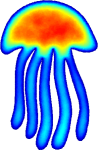 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
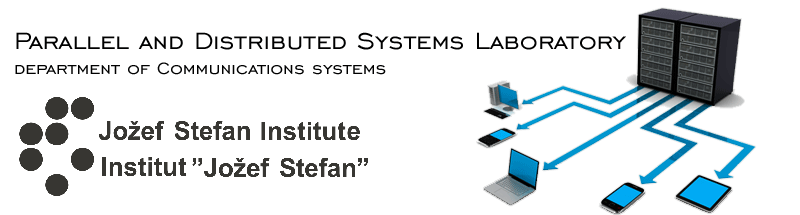 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_SHAPEUNION_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_SHAPEUNION_FWD_HPP_
27 template <
typename vec_t>
49 bool contains(
const vec_t& point)
const override {
50 return sh1->contains(point) ||
sh2->contains(point);
54 return sh1->hasContains() &&
sh2->hasContains();
57 std::pair<vec_t, vec_t>
bbox()
const override;
61 scalar_t step,
int internal_type,
int boundary_type)
const override;
63 const std::function<
scalar_t(vec_t)>& dr,
int type)
const override;
65 const std::function<
scalar_t(vec_t)>& dr,
int internal_type,
66 int boundary_type)
const override;
69 std::ostream&
print(std::ostream& os)
const override;
74 #endif // MEDUSA_BITS_DOMAINS_SHAPEUNION_FWD_HPP_
Root namespace for the whole library.
deep_copy_unique_ptr< DomainShape< vec_t > > sh1
First shape in the union.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
Class representing a union of two shapes.
DomainDiscretization< vec_t > discretizeWithDensity(const std::function< scalar_t(vec_t)> &dr, int internal_type, int boundary_type) const override
Returns a discretization of the domain with spatially variable step.
deep_copy_unique_ptr< DomainShape< vec_t > > sh2
Second shape in the union.
DomainDiscretization< vec_t > discretizeWithStep(scalar_t step, int internal_type, int boundary_type) const override
Returns a discretization of this shape with approximately uniform distance step between nodes.
ShapeUnion(const DomainShape< vec_t > &sh1, const DomainShape< vec_t > &sh2)
Construct a union of two shapes.
const DomainShape< vec_t > & first() const
Access the first shape.
DomainDiscretization< vec_t > discretizeBoundaryWithStep(scalar_t step, int type) const override
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
Base class for geometric shapes of domains.
bool hasContains() const override
Return true if shape has contains() method implemented.
const DomainShape< vec_t > & second() const
Access the second shape.
std::pair< vec_t, vec_t > bbox() const override
Return the bounding box of the domain.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const override
Discretizes boundary with given density and fill engine.
ShapeUnion< vec_t > * clone() const override
Polymorphic clone pattern.
bool contains(const vec_t &point) const override
Return true if point is not more than margin() outside the domain.
Unique pointer with polymorphic deep copy semantics.
vec_t::Scalar scalar_t
Scalar data type used in computation.
std::ostream & print(std::ostream &os) const override
Output information about this shape to given output stream os.