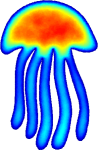 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
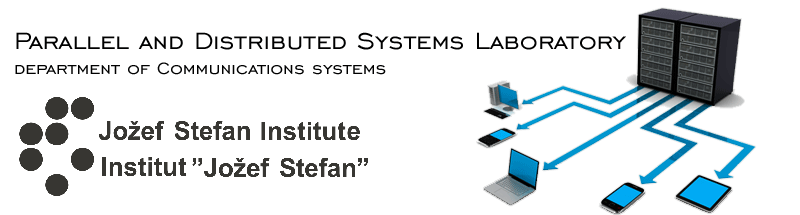 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_STLSHAPE_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_STLSHAPE_FWD_HPP_
29 template <
typename vec_t>
36 static_assert(
dim == 3,
"STL files are for 3D models only.");
38 std::vector<std::array<int, 3>>
faces_;
44 STLShape(
const std::vector<STL::Triangle>& stl);
51 bool contains(
const vec_t& )
const override {
return true; }
55 std::pair<vec_t, vec_t>
bbox()
const override {
return bbox_; }
59 const std::function<
scalar_t(vec_t)>&,
int)
const override;
61 std::ostream&
print(std::ostream& ostream)
const override {
62 return ostream <<
"STL shape with " <<
vertices_.size() <<
" vertices and "
63 <<
faces_.size() <<
" faces.";
73 return vec_t(p.
x, p.
y, p.
z);
79 #endif // MEDUSA_BITS_DOMAINS_STLSHAPE_FWD_HPP_
static vec_t v(const STL::Point &p)
Convert STL point to Vec3d.
Root namespace for the whole library.
Class representing domain discretization along with an associated shape.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &, int) const override
Discretizes boundary with given density and fill engine.
std::ostream & print(std::ostream &ostream) const override
Output information about this shape to given output stream os.
STLShape * clone() const override
Polymorphic clone pattern.
@ dim
Dimensionality of the domain.
std::pair< vec_t, vec_t > bbox_
Bounding box.
bool contains(const vec_t &) const override
Contains method is not supported and is approximated with a discrete version, which can be wrong.
Class representing an object given by the STL file.
Base class for geometric shapes of domains.
bool hasContains() const override
Return true if shape has contains() method implemented.
std::vector< vec_t > normals_
Normals for each face.
std::vector< std::array< int, 3 > > faces_
Faces, described with three indices of vertices.
STLShape(const std::vector< STL::Triangle > &stl)
Create STLShape from triangles read from STL file.
Holds one 3d Point in a STL file.
std::vector< vec_t > vertices_
3d vertices.
std::pair< vec_t, vec_t > bbox() const override
Return the bounding box of the domain.
vec_t::Scalar scalar_t
Scalar data type used in computation.