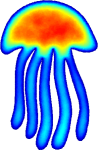 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
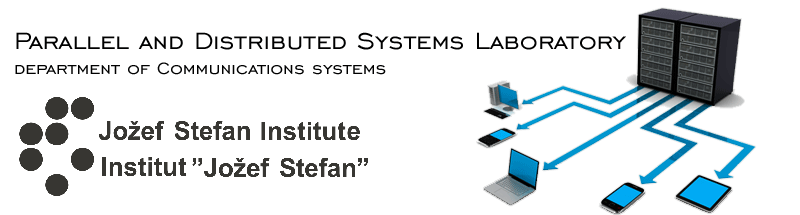 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_TYPES_RANGE_HPP_
2 #define MEDUSA_BITS_TYPES_RANGE_HPP_
14 template<
class T,
class Allocator>
16 this->resize(lst.size());
24 template<
class T,
class Allocator>
32 template<
class T,
class Allocator>
34 assert_msg(0 <= i && i < size(),
"Index %d out of range [%d, %d) when accessing Range "
35 "for write.", i, 0, size());
36 return std::vector<T>::operator[](i);
39 template<
class T,
class Allocator>
41 assert_msg(0 <= i && i < size(),
"Index %d out of range [%d, %d) when accessing Range "
42 "for read/write.", i, 0, size());
43 return std::vector<T>::operator[](i);
46 template<
class T,
class Allocator>
49 assert_msg(0 <= idx && idx < size(),
"Index %d out of range [%d, %d) when using "
50 "multiindexed read-write access.", idx, 0, size());
52 return {*
this, indexes};
55 template<
class T,
class Allocator>
59 assert_msg(0 <= idx && idx < size(),
"Index %d out of range [%d, %d) when using "
60 "multiindexed read access.", idx, 0, size());
62 return {*
this, indexes};
65 template<
class T,
class Allocator>
67 this->insert(this->end(), rng.begin(), rng.end());
71 template<
class T,
class Allocator>
74 ret.insert(ret.end(), rng.begin(), rng.end());
78 template<
class T,
class Allocator>
80 std::sort(indexes.begin(), indexes.end());
81 indexes.resize(std::unique(indexes.begin(), indexes.end()) - indexes.begin());
82 int sz = indexes.size();
83 auto it = std::vector<T, Allocator>::begin();
84 auto cur = std::vector<T, Allocator>::begin();
87 while (cur != std::vector<T, Allocator>::end()) {
88 if (to_remove < sz && c == indexes[to_remove]) {
91 *it++ = std::move(*cur);
96 std::vector<T, Allocator>::resize(it - std::vector<T, Allocator>::begin());
99 template<
class T,
class Allocator>
100 template<
class Predicate>
104 if (predicate(
operator[](i))) ret.push_back(i);
108 template<
class T,
class Allocator>
109 template<
typename UnaryOp>
111 Range<decltype(op(this->
operator[](0)))> r;
117 template<
class T,
class Allocator>
122 template<
class T,
class Allocator>
127 template<
class T,
class Allocator>
132 template<
class T,
class Allocator>
137 template<
class T,
class Allocator>
142 template<
class T,
class Allocator>
148 template <
class T,
class Allocator>
149 template <
typename V>
154 template <
class T,
class Allocator>
155 template <
typename V>
160 template <
class T,
class Allocator>
161 template <
typename V,
typename D>
163 assert_msg(step != 0,
"Step must be nonzero.");
164 assert_msg((stop - start) / step >= 0,
"Step %d must be in the direction from start %d to "
165 "stop %d.", step, start, stop);
166 Range<T> ret; ret.reserve((stop - start) / step);
167 for (V i = start; (step > 0) ? i < stop : i > stop; i += step) {
176 #endif // MEDUSA_BITS_TYPES_RANGE_HPP_
reference operator[](size_type i)
Overload vector's [] to assert parameter.
indexes_t filter(const Predicate &predicate) const
Returns list of indexes for which predicate returns true.
indexes_t operator>=(const value_type &v) const
Returns list of indexes of elements that are greater or equal to v.
Root namespace for the whole library.
indexes_t operator!=(const value_type &v) const
Returns list of indexes of elements that are not equal to v.
std::vector< value_t, std::allocator< value_t > >::const_reference const_reference
This container's const reference to value type.
indexes_t operator<=(const value_type &v) const
Returns list of indexes of elements that are less or equal to v.
std::vector< T, Allocator >::reference reference
This container's reference to value type.
auto map(UnaryOp op) -> Range< decltype(op(this->operator[](0)))> const
Returns a new range, obtained by applying op to all elements of this Range.
This class represents a non contiguous view to a Range, allowing for read and write operations.
indexes_t operator<(const value_type &v) const
Returns list of indexes of elements that are less than v.
static Range seq(V n)
Returns range {0, ..., n-1}.
indexes_t operator==(const value_type &v) const
Returns list of indexes of elements that are equal to v.
#define assert_msg(cond,...)
Assert with better error reporting.
mm::indexes_t filter(const Pred &pred) const
Returns list of indexes for which predicate returns true.
std::vector< int > indexes_t
Class representing a collection of indices.
std::vector< value_t, std::allocator< value_t > >::value_type value_type
This container's value type.
Range & operator=(const Range &o)=default
Default copy assignment.
int size_type
This container's size type.
indexes_t operator>(const value_type &v) const
Returns list of indexes of elements that are greater than v.
Range & append(const Range &rng)
Append all elements of rng to self.
Range join(const Range &rng) const
Return new copy containing this range's elements followed by all elements of rng.
container_t & sort(container_t &v)
Sorts a container inplace.
An extension of std::vector<T> to support additional useful operations.
This class represents a non contiguous view to a Range, allowing for read-only operations.
void remove(indexes_t indexes)
Remove elements wih given indexes.