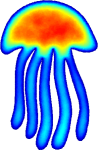 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
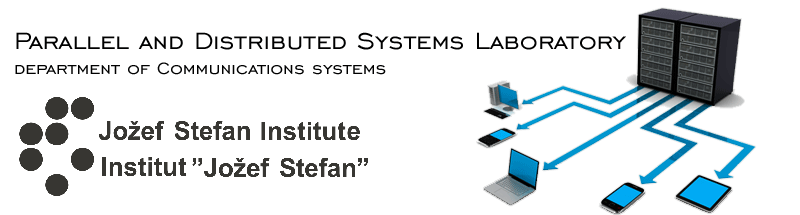 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_POLYGONSHAPE_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_POLYGONSHAPE_FWD_HPP_
26 template <
typename vec_t>
28 static_assert(
vec_t::dim == 2,
"Only available in 2 dimensions.");
62 bool contains(
const vec_t& point)
const override;
64 std::pair<vec_t, vec_t>
bbox()
const override;
79 const std::function<
scalar_t(vec_t)>& dr,
int type)
const override;
83 std::ostream&
print(std::ostream& os)
const override {
84 return os <<
"Polygon shape with " <<
points_.size() <<
" points.";
94 static inline scalar_t isLeft(
const vec_t& P0,
const vec_t& P1,
const vec_t& P2) {
95 return (P1[0] - P0[0]) * (P2[1] - P0[1]) - (P2[0] - P0[0]) * (P1[1] - P0[1]);
104 #endif // MEDUSA_BITS_DOMAINS_POLYGONSHAPE_FWD_HPP_
PolygonShape< vec_t > * clone() const override
Polymorphic clone pattern.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const override
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
DomainDiscretization< vec_t > discretizeBoundaryWithStep(scalar_t step, int type) const override
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
@ dim
Number of elements of this matrix.
Shape representing a simple (i.e. non self intersecting) nonempty polygon in 2D, which is given as a ...
@ dim
Dimensionality of the domain.
PolygonShape(const std::vector< vec_t > &points)
Create polygon given a sequence of points.
Base class for geometric shapes of domains.
std::vector< vec_t > points_with_margin_
Polygon extended by margin_, for contains checks.
vec_t vector_t
Vector data type used in computations.
virtual DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const =0
Discretizes boundary with given density and fill engine.
const std::vector< vec_t > & points() const
Get points representing the polygon.
std::vector< vec_t > points_
The points that define the polygon, stored in CCW order.
virtual DomainDiscretization< vec_t > discretizeBoundaryWithStep(scalar_t step, int type) const
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
void extendByMargin()
Save a version of points extended by margin_ for later contains checks.
bool contains(const vec_t &point) const override
Winding number test for point in a polygon inclusion (paying respect to margin).
scalar_t margin() const
Returns current margin.
void setMargin(scalar_t margin) override
Computes the polygon extended by margin as well.
std::pair< vec_t, vec_t > bbox() const override
Return the bounding box of the domain.
static scalar_t isLeft(const vec_t &P0, const vec_t &P1, const vec_t &P2)
Tests if a point is left, on, or right of an infinite line.
vec_t::Scalar scalar_t
Scalar data type used in computation.
std::ostream & print(std::ostream &os) const override
Output information about this shape to given output stream os.