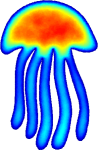 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
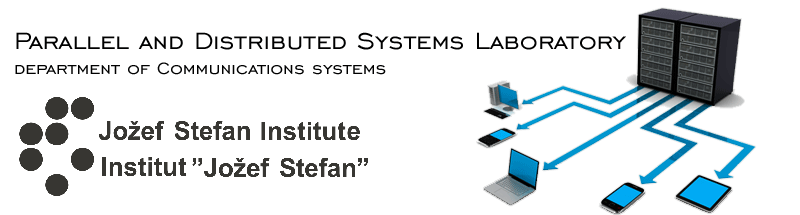 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_GENERALSURFACEFILL_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_GENERALSURFACEFILL_FWD_HPP_
16 template <
typename vec_t>
17 class DomainDiscretization;
19 template <
typename vec_t>
48 template <
typename vec_t,
typename param_vec_t>
104 template <
typename param_func_t,
typename jm_func_t,
typename search_t,
typename spacing_func_t>
106 const param_func_t& param_function,
const jm_func_t& param_jacobian,
107 const spacing_func_t& spacing_function,
108 search_t& tree,
int type = 0)
const;
111 template <
typename param_func_t,
typename search_t,
typename spacing_func_t>
113 const param_func_t& param,
const spacing_func_t& spacing_function,
114 search_t& tree,
int type = 0)
const;
117 template <
typename param_func_t,
typename jm_func_t,
typename spacing_func_t>
119 const param_func_t& param_function,
const jm_func_t& param_jacobian,
120 const spacing_func_t& spacing_function,
int type = 0)
const;
123 template <
typename param_func_t,
typename jm_func_t>
125 const param_func_t& param_function,
const jm_func_t& param_jacobian,
126 const scalar_t& h,
int type = 0)
const {
127 this->
operator()(domain, param_domain, param_function, param_jacobian,
128 [=] (
const vec_t&) {
return h; }, type);
132 template <
typename param_func_t,
typename jm_func_t,
typename spacing_func_t>
134 const param_func_t& param_function,
const jm_func_t& param_jacobian,
135 const spacing_func_t& spacing_function,
int type = 0)
const;
138 template <
typename param_func_t,
typename jm_func_t>
140 const param_func_t& param_function,
const jm_func_t& param_jacobian,
141 const scalar_t& h,
int type = 0)
const {
142 this->
operator()(domain, param_domain_shape, param_function, param_jacobian,
143 [=] (
const vec_t&) {
return h; }, type);
148 #endif // MEDUSA_BITS_DOMAINS_GENERALSURFACEFILL_FWD_HPP_
void operator()(domain_t &domain, param_domain_t ¶m_domain, const param_func_t ¶m_function, const jm_func_t ¶m_jacobian, const spacing_func_t &spacing_function, search_t &tree, int type=0) const
Fills the parametrically given surface with a quality node distribution according to the spacing_func...
Root namespace for the whole library.
void operator()(domain_t &domain, DomainShape< param_vec_t > ¶m_domain_shape, const param_func_t ¶m_function, const jm_func_t ¶m_jacobian, const scalar_t &h, int type=0) const
Overload for constant function and Shape instead of DomainDiscretization.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
@ dim
Number of elements of this matrix.
int max_points
Maximal number of points generated.
vec_t::scalar_t scalar_t
Scalar type.
int seed_
Seed for the random number generator.
Base class for geometric shapes of domains.
@ dim
Dimensionality of the domain.
int n_samples
Number of samples.
DomainDiscretization< param_vec_t > param_domain_t
Parametric domain discretization type.
void operator()(domain_t &domain, param_domain_t ¶m_domain, const param_func_t ¶m_function, const jm_func_t ¶m_jacobian, const scalar_t &h, int type=0) const
Overload for constant function.
DomainDiscretization< vec_t > domain_t
Domain discretization type.
scalar_t zeta
Proximity tolerance.
GeneralSurfaceFill & proximityTolerance(scalar_t zeta)
Set proximity tolerance.
param_vec_t::scalar_t param_scalar_t
Parametric domain scalar type.
void fillParametrization(domain_t &domain, param_domain_t ¶m_domain, const param_func_t ¶m, const spacing_func_t &spacing_function, search_t &tree, int type=0) const
Version with single function returning a pair of point and jacobian.
GeneralSurfaceFill & numSamples(int n_samples)
Controls the number of generated candidates from each point.
@ param_dim
Dimensionality of the parametric domain.
GeneralSurfaceFill & maxPoints(int max_points)
Maximal number of points generated.
Implements general n-d node placing algorithm for parametrically given d-d surfaces,...
GeneralSurfaceFill & seed(int seed)
Set custom seed for the random number generator.