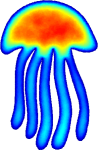 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
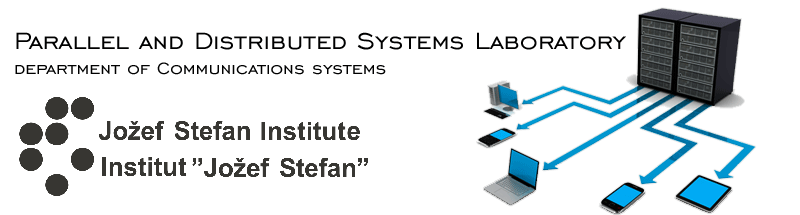 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_BOXSHAPE_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_BOXSHAPE_FWD_HPP_
27 template <
typename vec_t>
54 bool contains(
const vec_t& point)
const override;
56 std::pair<vec_t, vec_t>
bbox()
const override {
return {
beg_,
end_}; }
60 scalar_t step,
int internal_type,
int boundary_type)
const override;
62 const std::function<
scalar_t(vec_t)>& dr,
int type)
const override;
90 int boundary_type = 0)
const;
94 std::ostream&
print(std::ostream& os)
const override;
99 #endif // MEDUSA_BITS_DOMAINS_BOXSHAPE_FWD_HPP_
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
Class representing domain discretization along with an associated shape.
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
const vec_t & end() const
Returns the second point defining the box.
const vec_t & beg() const
Returns the first point defining the box.
DomainDiscretization< vec_t > discretizeBoundary(const Vec< int, dim > &counts, int type=0) const
Uniformly discretizes underlying domain boundary.
Base class for geometric shapes of domains.
BoxShape(vec_t beg, vec_t end)
Constructs a n dimensional box shaped domain defined by two n dimensional points - vertices at ends o...
vec_t end_
Larger of the edge points.
bool contains(const vec_t &point) const override
Return true if point is not more than margin() outside the domain.
DomainDiscretization< vec_t > discretizeBoundaryWithDensity(const std::function< scalar_t(vec_t)> &dr, int type) const override
Discretizes boundary with given density and fill engine.
Class for working with box shaped domains.
DomainDiscretization< vec_t > discretizeWithStep(scalar_t step, int internal_type, int boundary_type) const override
Returns a discretization of this shape with approximately uniform distance step between nodes.
DomainDiscretization< vec_t > discretizeBoundaryWithStep(scalar_t step, int type) const override
Returns a discretization of the boundary of this shape with approximately uniform distance step betwe...
BoxShape< vec_t > * clone() const override
Polymorphic clone pattern.
DomainDiscretization< vec_t > discretize(const Vec< int, dim > &counts, int internal_type=0, int boundary_type=0) const
Uniformly discretizes underlying domain.
std::pair< vec_t, vec_t > bbox() const override
Return the bounding box of the domain.
std::ostream & print(std::ostream &os) const override
Output information about this shape to given output stream os.
vec_t::Scalar scalar_t
Scalar data type used in computation.
vec_t beg_
Smaller of the edge points.