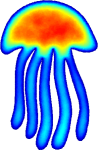 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
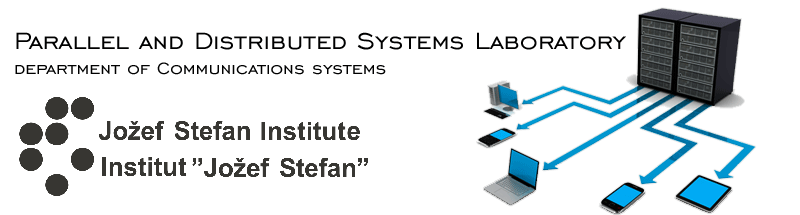 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_OPERATORS_SHAPE_FLAGS_HPP_
2 #define MEDUSA_BITS_OPERATORS_SHAPE_FLAGS_HPP_
34 if (f &
sh::d1) s +=
" d1";
36 if (f &
sh::d2) s +=
" d2";
41 template <shape_flags mask,
int dim>
43 static_assert(mask < 0,
"Type for this mask not defined");
48 template <
int dim>
struct operator_tuple<
lap,
dim> {
typedef std::tuple<Lap<dim>>
type; };
49 template <
int dim>
struct operator_tuple<
lap|
d1,
dim> {
typedef std::tuple<Lap<dim>, Der1s<dim>>
type; };
50 template <
int dim>
struct operator_tuple<
lap|
d2,
dim> {
typedef std::tuple<Lap<dim>, Der2s<dim>>
type; };
51 template <
int dim>
struct operator_tuple<
lap|
d1|
d2,
dim> {
typedef std::tuple<Lap<dim>, Der1s<dim>, Der2s<dim>>
type; };
52 template <
int dim>
struct operator_tuple<
d1,
dim> {
typedef std::tuple<Der1s<dim>>
type; };
53 template <
int dim>
struct operator_tuple<
d1|
d2,
dim> {
typedef std::tuple<Der1s<dim>, Der2s<dim>>
type; };
54 template <
int dim>
struct operator_tuple<
d2,
dim> {
typedef std::tuple<Der2s<dim>>
type; };
61 #endif // MEDUSA_BITS_OPERATORS_SHAPE_FLAGS_HPP_
static const shape_flags d1
Indicates to calculate d1 shapes.
static const shape_flags div
Indicates to prepare all shapes needed for div.
Root namespace for the whole library.
static const shape_flags graddiv
Indicates to prepare all shapes needed for graddiv.
static const shape_flags lap
Indicates to calculate laplace shapes.
@ dim
Number of elements of this matrix.
unsigned int shape_flags
Type representing flags for shape functions.
std::string str(shape_flags f)
Convert shape flags to a string representation.
static const shape_flags d2
Indicates to calculate d2 shapes.
static const shape_flags all
Indicates to prepare all shapes, default.
void type
The type of the operator tuple.
static const shape_flags grad
Indicates to prepare all shapes needed for grad.
Converts shape mask to operator tuple type.