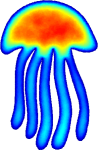 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
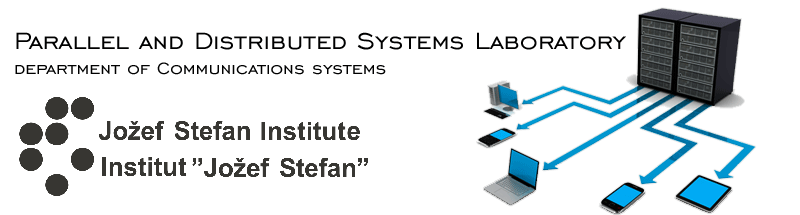 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_TYPES_MATRIXBASEADDONS_HPP_
2 #define MEDUSA_BITS_TYPES_MATRIXBASEADDONS_HPP_
14 dim = RowsAtCompileTime * ColsAtCompileTime };
19 template<
typename OtherDerived>
20 bool operator<(
const MatrixBase<OtherDerived>& arg)
const {
21 for (
int i = 0; i < this->size(); ++i) {
22 if (this->
operator[](i) == arg[i])
continue;
23 return this->operator[](i) < arg[i];
29 template<
typename OtherDerived>
30 bool operator>(
const MatrixBase<OtherDerived>& arg)
const {
return arg < *
this; }
33 template<
typename OtherDerived>
34 bool operator<=(
const MatrixBase<OtherDerived>& arg)
const {
return !(arg < *
this); }
37 template<
typename OtherDerived>
38 bool operator>=(
const MatrixBase<OtherDerived>& arg)
const {
return !(*
this < arg); }
48 for (
int i = 0; i < size(); ++i)
49 if (pred(this->
operator[](i))) ret.push_back(i);
56 for (
int i = 0; i < size(); ++i)
57 if (this->
operator[](i) < v) ret.push_back(i);
64 for (
int i = 0; i < size(); ++i)
65 if (this->
operator[](i) > v) ret.push_back(i);
72 for (
int i = 0; i < size(); ++i)
73 if (this->
operator[](i) <= v) ret.push_back(i);
80 for (
int i = 0; i < size(); ++i)
81 if (this->
operator[](i) >= v) ret.push_back(i);
88 for (
int i = 0; i < size(); ++i)
89 if (this->
operator[](i) == v) ret.push_back(i);
96 for (
int i = 0; i < size(); ++i)
97 if (this->
operator[](i) != v) ret.push_back(i);
101 #endif // MEDUSA_BITS_TYPES_MATRIXBASEADDONS_HPP_
Scalar scalar_t
Type of the elements, alias of Scalar.
bool operator>(const MatrixBase< OtherDerived > &arg) const
Lexicographical compare of vectors.
@ dim
Number of elements of this matrix.
bool operator<(const MatrixBase< OtherDerived > &arg) const
Lexicographical compare of vectors.
bool operator>=(const MatrixBase< OtherDerived > &arg) const
Lexicographical compare of vectors.
mm::indexes_t filter(const Pred &pred) const
Returns list of indexes for which predicate returns true.
mm::indexes_t operator!=(const Scalar &v) const
Returns list of indexes for which their elements compare not equal to a.
std::vector< int > indexes_t
Class representing a collection of indices.
mm::indexes_t operator==(const Scalar &v) const
Returns list of indexes for which their elements compare equal to a.
bool operator<=(const MatrixBase< OtherDerived > &arg) const
Lexicographical compare of vectors.