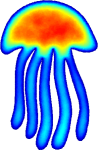 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
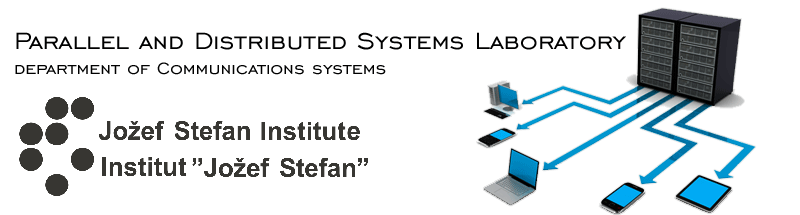 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_UTILS_NUMUTILS_HPP_
2 #define MEDUSA_BITS_UTILS_NUMUTILS_HPP_
27 int iceil(T x) {
return static_cast<int>(std::ceil(x)); }
36 int ifloor(T x) {
return static_cast<int>(std::floor(x)); }
39 template <
unsigned int exponent>
40 inline double ipow(
double base) {
41 return ipow<exponent - 1>(base) * base;
46 inline double ipow<0>(
double) {
return 1; }
56 inline T
ipow(T b,
int e) {
73 if (e < 0)
return 1.0 /
ipow(b, -e);
74 else return ipow(b, e);
79 inline constexpr
int signum(T x, std::false_type) {
84 inline constexpr
int signum(T x, std::true_type) {
85 return (T(0) < x) - (x < T(0));
95 return signum(x, std::is_signed<T>());
121 for (
int i =
dim - 1; i >= 0; --i) {
122 if (counter[i] >= limit[i] - 1) {
144 for (
int i =
dim - 1; i >= 0; --i) {
145 if (counter[i] >= high[i] - 1) {
184 for (
int i =
dim - 1; i >= 0; --i) {
185 if (counter[i] == high[i] - 1 || (high[i] == 0 && counter[i] == size[i] - 1)) {
189 if (counter[i] >= size[i]) counter[i] = 0;
209 template <
class scalar_t,
int dim>
214 for (
int i = 0; i <
dim; ++i) {
215 if (include_boundary[i]) assert(counts[i] >= 2);
216 else assert(counts[i] >= 0);
217 if (counts[i] == 0)
return ret;
220 for (
int i = 0; i <
dim; ++i) {
221 if (include_boundary[i]) step[i] = (end[i] - beg[i]) / (counts[i] - 1);
222 else step[i] = (end[i] - beg[i]) / (counts[i] + 1);
227 for (
int i = 0; i <
dim; ++i) {
228 if (include_boundary[i]) tmp[i] = beg[i] + counter[i] * step[i];
229 else tmp[i] = beg[i] + (counter[i] + 1) * step[i];
237 template <
class scalar_t,
int dim>
244 template <
typename scalar_t>
247 if (include_boundary) {
248 assert_msg(count >= 2,
"Count must be >= 2, got %d.", count);
249 scalar_t step = (end - beg) / (count - 1);
250 for (
int i = 0; i < count; ++i) {
251 ret.push_back(beg + step * i);
254 assert_msg(count >= 0,
"Count must be >= 0, got %d.", count);
255 scalar_t step = (end - beg) / (count + 1);
256 for (
int i = 1; i <= count; ++i) {
257 ret.push_back(beg + step * i);
278 template<
typename function_t,
typename input_t,
typename output_t,
bool verbose = false>
279 input_t
bisection(
const function_t& f, input_t lo, input_t hi, output_t target = 0.0,
280 input_t tolerance = 1e-4,
int max_iter = 40) {
281 input_t a = lo, d = hi - lo;
282 output_t fa = f(lo) - target;
283 output_t fb = f(hi) - target;
286 "Function values have the same sign, f(a) = %s, f(b) = %s.", fa, fb);
288 while (d > tolerance) {
289 d /=
static_cast<input_t
>(2.);
290 output_t fc = f(a+d) - target;
292 if (verbose) std::cout <<
"f(" << a+d <<
")=" << fc + target <<
"\n";
299 std::cerr <<
"\nBisection ended without finding the solution after max_num"
300 " iterations." << std::endl;
309 #endif // MEDUSA_BITS_UTILS_NUMUTILS_HPP_
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
bool incrementCyclicCounter(Vec< int, dim > &counter, const Vec< int, dim > &low, const Vec< int, dim > &high, const Vec< int, dim > &size)
Increments a multi-dimensional counter with given upper and lower limits and global upper size,...
Eigen::Matrix< scalar_t, dim, 1, Eigen::ColMajor|Eigen::AutoAlign, dim, 1 > Vec
Fixed size vector type, representing a mathematical 1d/2d/3d vector.
T ipowneg(T b, int e)
Compute possibly negative integer power b^e.
@ dim
Number of elements of this matrix.
double ipow(double base)
Compile time integer power, returns base raised to power exponent.
input_t bisection(const function_t &f, input_t lo, input_t hi, output_t target=0.0, input_t tolerance=1e-4, int max_iter=40)
Solves f(x) = target using bisection.
#define assert_msg(cond,...)
Assert with better error reporting.
int ifloor(T x)
Floors a floating point to an integer.
int iceil(T x)
Ceils a floating point to an integer.
Range< Vec< scalar_t, dim > > linspace(const Vec< scalar_t, dim > &beg, const Vec< scalar_t, dim > &end, const Vec< int, dim > &counts, const Vec< bool, dim > include_boundary=true)
Multidimensional clone of Matlab's linspace function.
double ipow< 0 >(double)
Compile time integer power (base case 0)
bool incrementCounter(Vec< int, dim > &counter, const Vec< int, dim > &limit)
Increments a multi-dimensional counter with given limits.
constexpr int signum(T x, std::false_type)
Signum overload for unsigned types.
An extension of std::vector<T> to support additional useful operations.