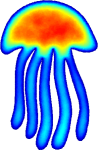 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
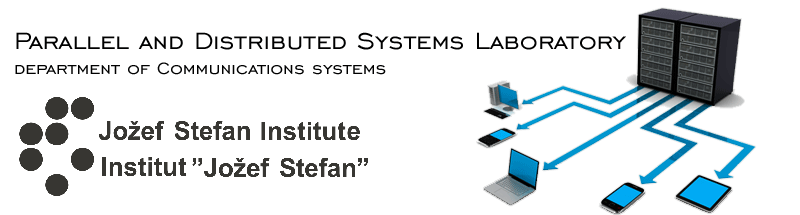 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_IO_XML_FWD_HPP_
2 #define MEDUSA_BITS_IO_XML_FWD_HPP_
15 #include <rapidxml/rapidxml.hpp>
42 rapidxml::xml_document<char>
doc;
54 explicit XML(
const std::string& filename);
59 void load(
const std::string& filename);
76 char*
getString(
const std::vector<std::string>& path,
const std::string& attribute_name)
const;
78 void setString(
const std::vector<std::string>& path,
const std::string& attribute_name,
79 const std::string& content);
87 void getAllRecursive(
const rapidxml::xml_node<>* node, std::string path,
88 std::vector<std::pair<std::string, std::string>>& all_attr)
const;
94 static std::pair<std::vector<std::string>, std::string>
splitPath(std::string path);
97 static std::string
join(
const std::vector<std::string>& path);
101 bool exists(
const std::string& path)
const;
111 template <
typename T>
112 T
get(
const std::string& path)
const;
126 template <
typename T>
127 void set(
const std::string& path,
const T& value,
bool overwrite =
false);
130 std::vector<std::pair<std::string, std::string>>
getAll()
const;
135 const rapidxml::xml_document<char>&
documentRoot()
const;
138 friend std::ostream&
operator<<(std::ostream& os,
const XML& xml);
144 #endif // MEDUSA_BITS_IO_XML_FWD_HPP_
Root namespace for the whole library.
T get(const std::string &path) const
Reads a values from an attribute specified by path.
void loadFromStoredContents()
Loads the XML document from a stored null-terminated file_contents.
std::vector< std::pair< std::string, std::string > > getAll() const
Returns all pairs (path, attribute_value).
XML()=default
Creates an XML reader linked to no document.
void loadFileHelper(const std::string &file)
Function for opening files, called by XML::XML(const std::string&) and XML::load().
char * getString(const std::vector< std::string > &path, const std::string &attribute_name) const
Reads the contents of the attribute specified by path as a string.
void getAllRecursive(const rapidxml::xml_node<> *node, std::string path, std::vector< std::pair< std::string, std::string >> &all_attr) const
Fills the all_attr array with all pairs (path, value) pairs that are descendants of node node at path...
void set(const std::string &path, const T &value, bool overwrite=false)
Saves a value to the attribute pointed to by path.
rapidxml::xml_document< char > & documentRoot()
Access to underlying XML root element from RapidXml library.
static std::pair< std::vector< std::string >, std::string > splitPath(std::string path)
Splits dot separated path into elements path and attribute name.
Class for reading and storing values to XML files.
void setString(const std::vector< std::string > &path, const std::string &attribute_name, const std::string &content)
Writes the contents of string content to the attribute specified by path.
std::vector< char > file_contents
Whole XML file contents.
bool exists(const std::string &path) const
Returns true if the an attribute specified by path exists and false otherwise.
rapidxml::xml_document< char > doc
XML document root.
friend std::ostream & operator<<(std::ostream &os, const XML &xml)
Prints the contents of currently loaded XML document.
static std::string join(const std::vector< std::string > &path)
Join a path into dot separated string.
void load(const std::string &filename)
Loads XML document from a file given by filename.