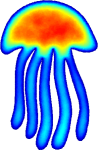 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
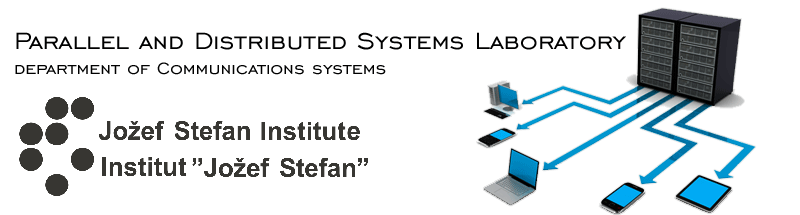 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_APPROXIMATIONS_WEIGHTFUNCTION_FWD_HPP_
2 #define MEDUSA_BITS_APPROXIMATIONS_WEIGHTFUNCTION_FWD_HPP_
24 template <
class vec_t>
44 return os <<
"NoWeight " << m.
dim <<
"D";
62 template <
class RBFType,
class vec_t>
64 static_assert(std::is_same<typename vec_t::scalar_t, typename RBFType::scalar_t>::value,
65 "Basis and underlying RBF must have the same scalar type.");
67 typedef RBFType
rbf_t;
82 template <
typename ...Args>
95 template <
typename V,
typename R>
101 template <
typename S>
class Gaussian;
119 #endif // MEDUSA_BITS_APPROXIMATIONS_WEIGHTFUNCTION_FWD_HPP_
vec_t vector_t
Vector type.
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
RBFWeight(const rbf_t &rbf)
Construct a weight from given RBF.
const rbf_t & rbf() const
Returns underlying RBF object.
Inverse Multiquadric Radial Basis Function.
@ dim
Number of elements of this matrix.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
Class representing no weight function, i.e. a constant 1.
friend std::ostream & operator<<(std::ostream &os, const NoWeight< V > &m)
Output basic info about given weight function.
vector_t::scalar_t scalar_t
Scalar type.
scalar_t operator()(const vector_t &point) const
Evaluate weight function at point.
scalar_t operator()(const vector_t &) const
Evaluate weight function at point. Returns 1.
RBFWeight(Args &&... args)
Perfect forwarding constructor. Construct RBFWeight as if you were constructing given RBF.
RBFType rbf_t
Radial basis function type.
@ dim
Dimensionality of the function domain.
Multiquadric Radial Basis Function.
Polyharmonic Radial Basis Function of odd order.
Represents a weight function constructed from a Radial Basis function.
RBFWeight()
Construct a weight with default construction of RBF.
@ dim
Dimensionality of the function domain.
friend std::ostream & operator<<(std::ostream &os, const RBFWeight< V, R > &m)
Output basic info about given weight function.
rbf_t & rbf()
Returns modifiable underlying RBF object.
vector_t::scalar_t scalar_t
Scalar type.
vec_t vector_t
Vector type.