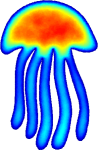 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
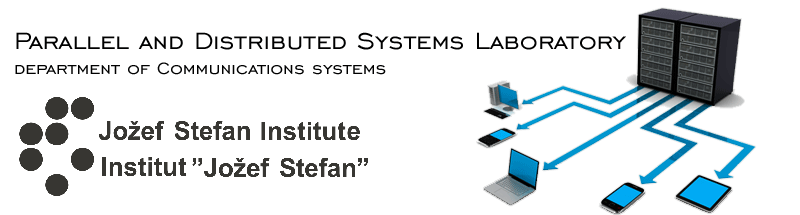 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_APPROXIMATIONS_SCALEFUNCTION_HPP_
2 #define MEDUSA_BITS_APPROXIMATIONS_SCALEFUNCTION_HPP_
26 template<
typename vec_t>
28 const std::vector<vec_t>& support) {
29 return (p - support[1]).norm();
33 return os <<
"ScaleToClosest";
46 template<
typename vec_t>
48 const std::vector<vec_t>& support) {
49 return (p - support.back()).norm();
53 return os <<
"ScaleToFarthest";
65 template<
typename vec_t>
68 const vec_t& ,
const std::vector<vec_t>& ) {
return 1.0; }
71 return os <<
"NoScale";
77 #endif // MEDUSA_BITS_APPROXIMATIONS_SCALEFUNCTION_HPP_
Root namespace for the whole library.
static vec_t::scalar_t scale(const vec_t &p, const std::vector< vec_t > &support)
Returns local scale of given point and its support.
Scalar scalar_t
Type of the elements, alias of Scalar.
friend std::ostream & operator<<(std::ostream &os, const ScaleToClosest &)
Print information about this scale function.
Scale function that scales to the closest neighbor.
friend std::ostream & operator<<(std::ostream &os, const NoScale &)
Print information about this scale function.
friend std::ostream & operator<<(std::ostream &os, const ScaleToFarthest &)
Print information about this scale function.
Scale function that scales to the farthest neighbor.
Scale function that indicates no scaling is performed.
static vec_t::scalar_t scale(const vec_t &p, const std::vector< vec_t > &support)
Returns local scale of given point and its support.
static vec_t::scalar_t scale(const vec_t &, const std::vector< vec_t > &)
Returns local scale of given point and its support.