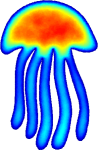 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
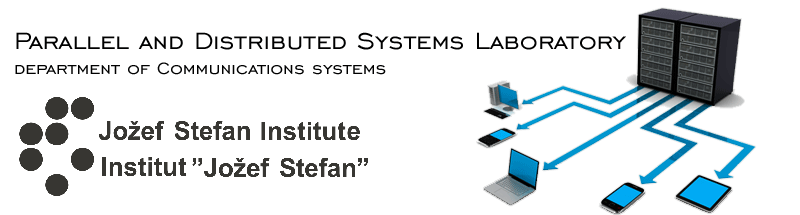 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_TYPES_SCALARFIELD_FWD_HPP_
2 #define MEDUSA_BITS_TYPES_SCALARFIELD_FWD_HPP_
26 template <
typename Scalar>
30 typedef Eigen::Matrix<Scalar, Eigen::Dynamic, 1>
Base;
32 using Base::operator=;
34 template <
typename OtherDerived>
35 ScalarField(
const Eigen::MatrixBase<OtherDerived>& other);
37 template <
typename OtherDerived>
54 template <
typename OtherDerived>
55 void operator=(
const Eigen::MatrixBase<OtherDerived>& other) {
65 using Base::operator[];
82 #endif // MEDUSA_BITS_TYPES_SCALARFIELD_FWD_HPP_
Root namespace for the whole library.
ScalarFieldView(ScalarField &sf, const indexes_t &indexes)
Construct a non contiguous view to a scalar field.
ScalarField & operator=(const Eigen::MatrixBase< OtherDerived > &other)
This method allows assignments of Eigen expressions to ScalarField.
Represents a discretization of a scalar field, a finite collection of scalars.
ScalarField(const Eigen::MatrixBase< OtherDerived > &other)
This constructor allows construction of ScalarField from Eigen expressions.
const indexes_t & indexes
Indexes of this view.
Eigen::Matrix< Scalar, Eigen::Dynamic, 1 > Base
Base class typedef.
Represents a non contiguous view to a scalar field.
T type
Underlying scalar type.
ScalarField< double > ScalarFieldd
Convenience typedef for ScalarField of doubles.
std::vector< int > indexes_t
Class representing a collection of indices.
void operator=(const scalar_t &s)
Assign a scalar to this view.
void operator=(const Eigen::MatrixBase< OtherDerived > &other)
Assign another Eigen expression to this view.
ScalarField & sf
Reference to the viewed field.
ScalarFieldView operator[](const indexes_t &indexes)
Multiindex view to given ScalarField.
Matrix(const Scalar &s)
Construct matrix from scalar. Enabled only for fixed size matrices.
Type trait for scalar fields to obtain their underlying scalar type.
Scalar scalar_t
Scalar data type.
ScalarField< float > ScalarFieldf
Convenience typedef for ScalarField of floats.
void operator=(const ScalarFieldView &other)
Assign another ScalarFieldView.